API Collection
Here is the collection of all the Tellephant API requests.
Get Postman Collection
Using these boilerplate requests, you can test and explore the APIs in any environment.
Messaging Service APIs
Template Messages
Template Messages Example request:
curl -X POST \
"https://api.tellephant.com/v1/send-message" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","to":919999999999,"channels":["whatsapp"],"whatsapp":{"contentType":"template","template":{"templateId":"dummy_template_name","language":"en","components":[]}}}'
const url = new URL(
"https://api.tellephant.com/v1/send-message"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
}
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/send-message',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'ABCDEFGHIJKLMNOPQRSTUVWXYZ',
'to' => 919999999999,
'channels' => [
'whatsapp',
],
'whatsapp' => [
'contentType' => 'template',
'template' => [
'templateId' => 'dummy_template_name',
'language' => 'en',
'components' => [],
],
],
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/send-message'
payload = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example Component Template (TEXT):
"components" : [
{
"type" : "body",
"parameters" : [
{
"type" : "text",
"text" : "Dummy Text 1"
},
{
"type" : "text",
"text" : "Dummy Text 2"
}
]
}
]
Example Component Template -TEXT (without any variables in text):
"components" : []
1Example Component Template (IMAGE):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - IMAGE (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
}
]
Example Component Template (DOCUMENT):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - DOCUMENT (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
}
]
Example Component Template (VIDEO):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - VIDEO (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
}
]
Example Component Template (Location):
"components": [
{
"type": "header",
"parameters": [
{
"type": "location",
"location": {
"latitude": 51.5005765,
"longitude": 7.4954884
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Your text here"
}
]
}
]
Example response (200):
{
"success": true,
"messageId": "CAGHDVFHVFUDUYRFGUFJYR"
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (401):
{
"status": false,
"error": "Please subscribe to addon first"
}
Example response (403):
{
"status": false,
"error": "Balance Low! Please recharge!"
}
Send messages via this path.
POST v1/send-message
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
to |
integer | required | Number to send the message |
channels |
array | required | Channel(s) to be used. |
whatsapp.contentType |
String | required | Content type used |
whatsapp.template |
array | required | Template Details |
whatsapp.template.templateId |
String | required | Template identifier string |
whatsapp.template.language |
String | required | Language in the template |
whatsapp.template.components |
array | required | Template Data |
Template (Text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
body | array | required | Parameters to be used in Component |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
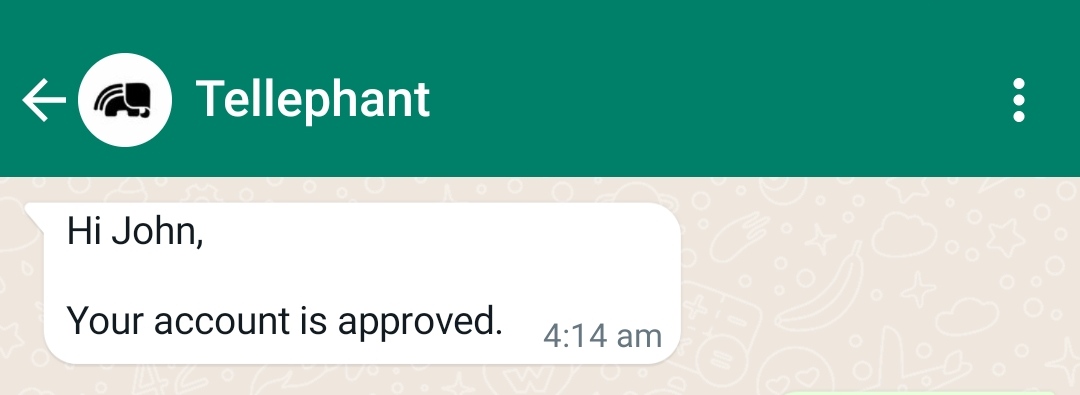
Template - Text (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
components |
body | array | present | Empty array of components |
Template (Image)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Image header |
url |
media | String | required | The url of the location where the image is stored |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
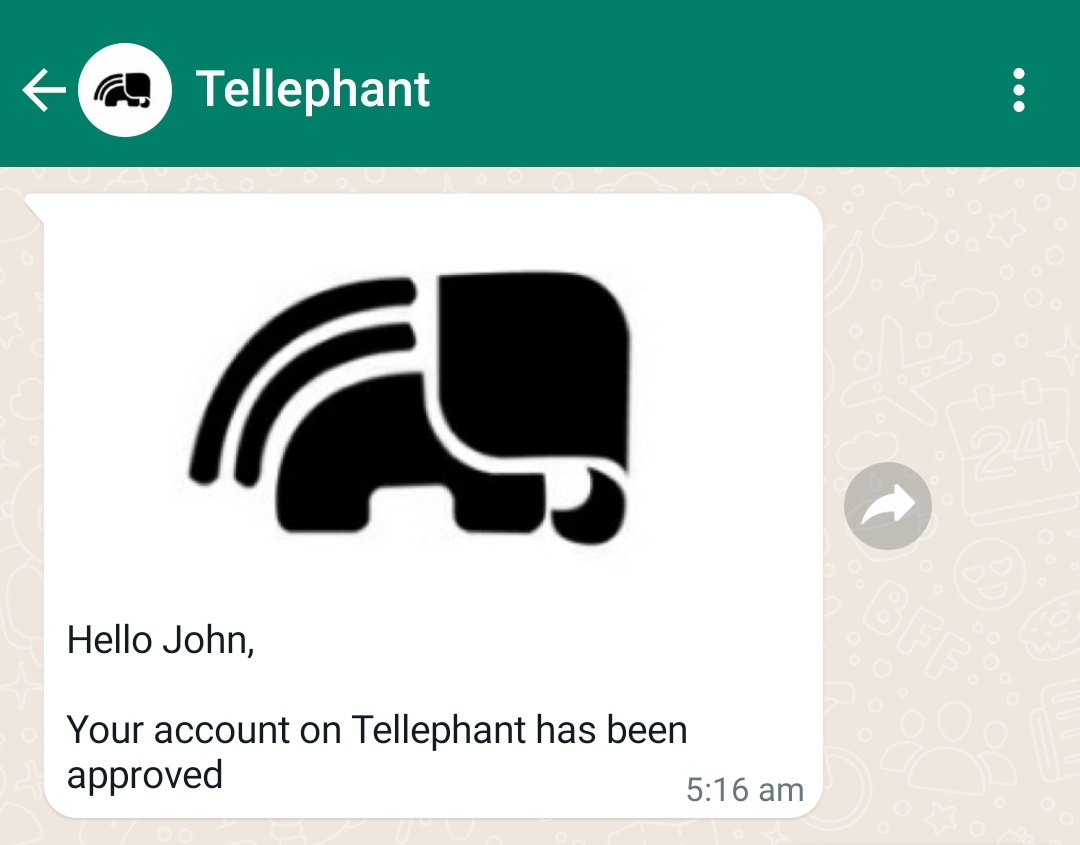
Template - Image (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Image header |
url |
media | String | required | The url of the location where the image is stored |
Template (Document)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Document header |
url |
media | String | required | The url of the location where the document is stored |
filename |
media | String | required | Document Name |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
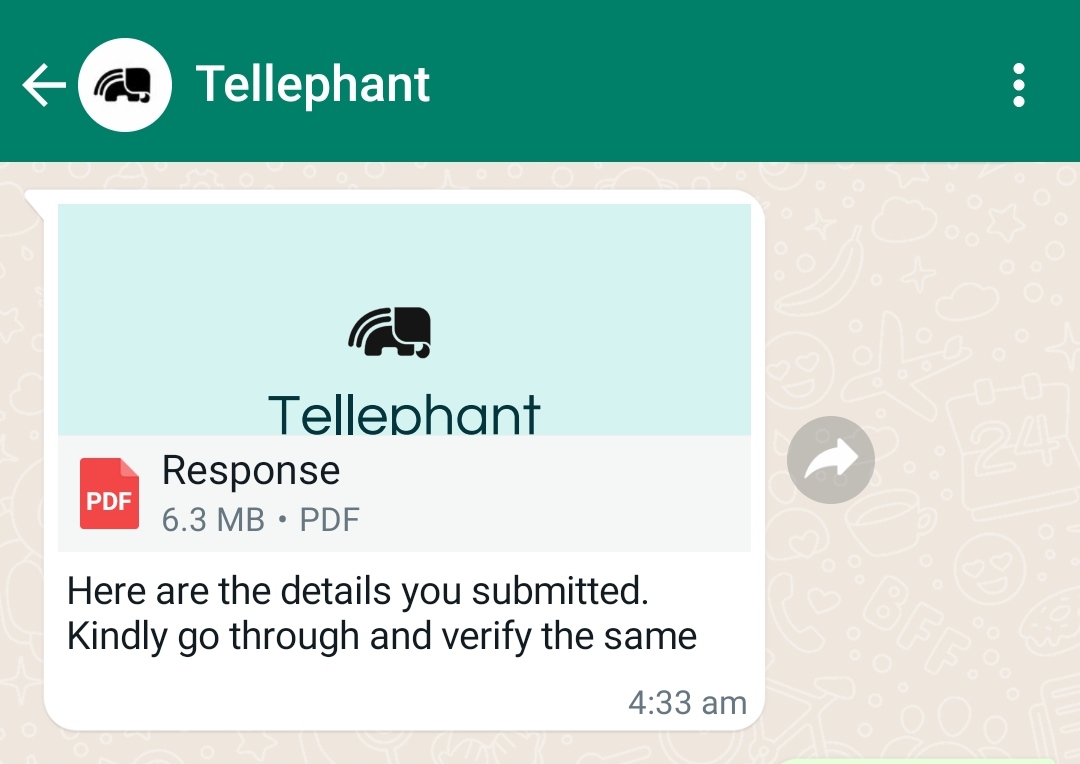
Template - Document (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Document header |
url |
media | String | required | The url of the location where the document is stored |
filename |
media | String | required | Document Name |
Template (VIDEO)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Video header |
url |
media | String | required | The url of the location where the video is stored |
caption |
media | String | optional | Additional caption for the video. |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
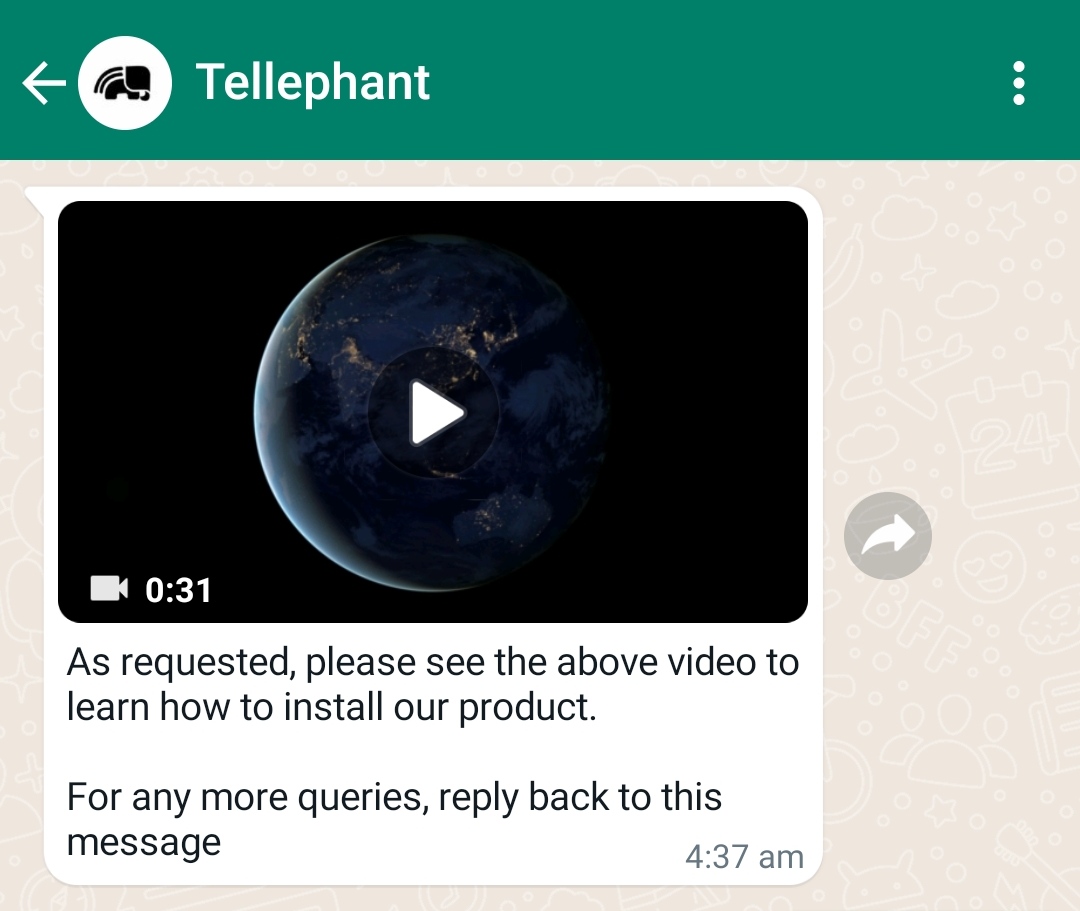
Template - VIDEO (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Video header |
url |
media | String | required | The url of the location where the video is stored |
caption |
media | String | optional | Additional caption for the video. |
Template (LOCATION)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Location component for WhatsApp Template |
latitude |
location | number(double) | required | Latitude component of location |
longitude |
location | number(double) | required | Longitude component of location |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
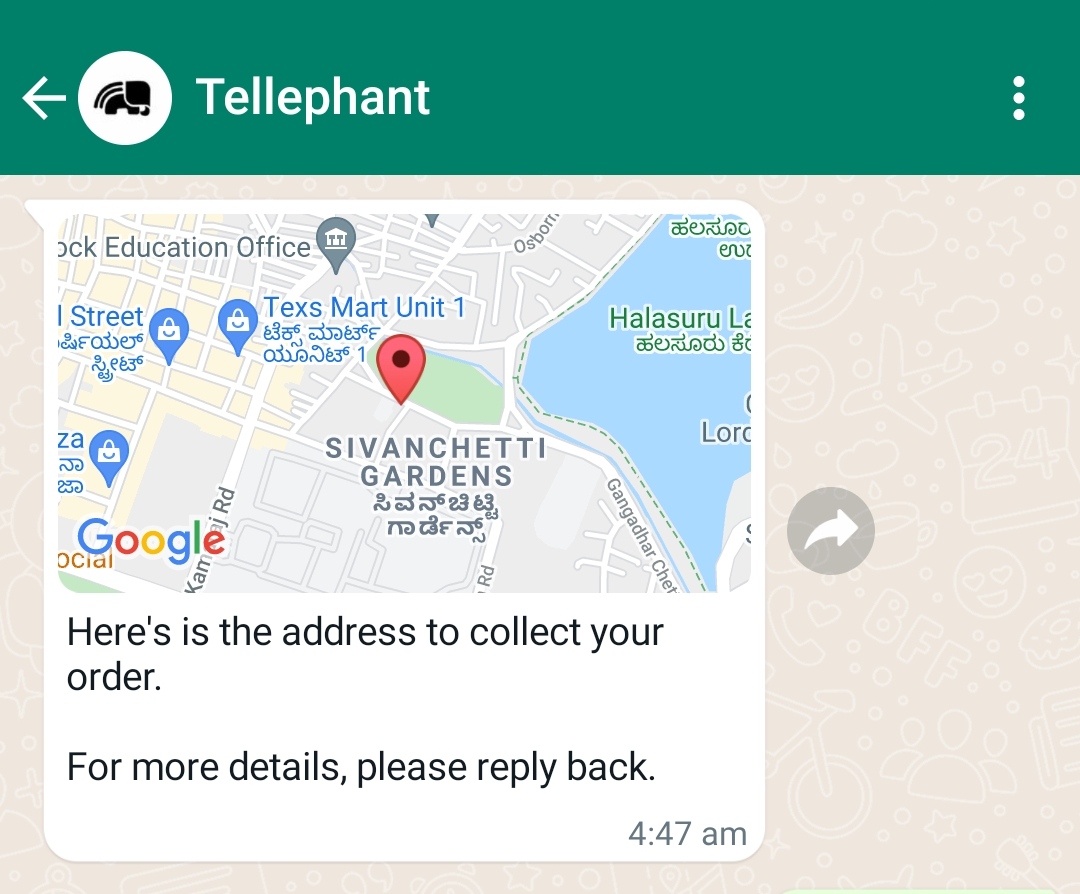
Template (Quick Reply Buttons)
Template Messages Example request:
curl -X POST \
"https://api.tellephant.com/v1/send-message" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","to":919999999999,"channels":["whatsapp"],"whatsapp":{"contentType":"template","template":{"templateId":"dummy_template_name","language":"en","components":[]}}}'
const url = new URL(
"https://api.tellephant.com/v1/send-message"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
}
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/send-message',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'ABCDEFGHIJKLMNOPQRSTUVWXYZ',
'to' => 919999999999,
'channels' => [
'whatsapp',
],
'whatsapp' => [
'contentType' => 'template',
'template' => [
'templateId' => 'dummy_template_name',
'language' => 'en',
'components' => [],
],
],
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/send-message'
payload = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example Component Template (TEXT with Quick Reply buttons):
"components" : [
{
"type" : "body",
"parameters" : [
{
"type" : "text",
"text" : "Dummy Text 1"
},
{
"type" : "text",
"text" : "Dummy Text 2"
}
]
}
]
Example Component Template - Static TEXT with Quick Reply buttons (without any variables in text):
"components" : []
1Example Component Template (IMAGE with Quick Reply buttons):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - IMAGE with Static TEXT and Quick Reply buttons (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
}
]
Example Component Template (DOCUMENT with Quick Reply buttons):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - DOCUMENT with Static TEXT and Quick Reply buttons (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
}
]
Example Component Template (VIDEO with Quick Reply buttons):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - VIDEO with Static TEXT and Quick Reply buttons (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
}
]
Example Component Template (Location with Quick Reply buttons):
"components": [
{
"type": "header",
"parameters": [
{
"type": "location",
"location": {
"latitude": 51.5005765,
"longitude": 7.4954884
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Your text here"
}
]
}
]
Example response (200):
{
"success": true,
"messageId": "CAGHDVFHVFUDUYRFGUFJYR"
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (401):
{
"status": false,
"error": "Please subscribe to addon first"
}
Example response (403):
{
"status": false,
"error": "Balance Low! Please recharge!"
}
Send messages via this path.
POST v1/send-message
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
to |
integer | required | Number to send the message |
channels |
array | required | Channel(s) to be used. |
whatsapp.contentType |
String | required | Content type used |
whatsapp.template |
array | required | Template Details |
whatsapp.template.templateId |
String | required | Template identifier string |
whatsapp.template.language |
String | required | Language in the template |
whatsapp.template.components |
array | required | Template Data |
Note-
Quick Replies are typically used if you wish to give your users the choice between 1–3 possible answers.
The user then simply needs to tap one of the quick replies to answer to your message.
No extra component is required in the request to send a Template with Quick Reply buttons.
Template (Text with Quick Reply buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
body | array | required | Parameters to be used in Component |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
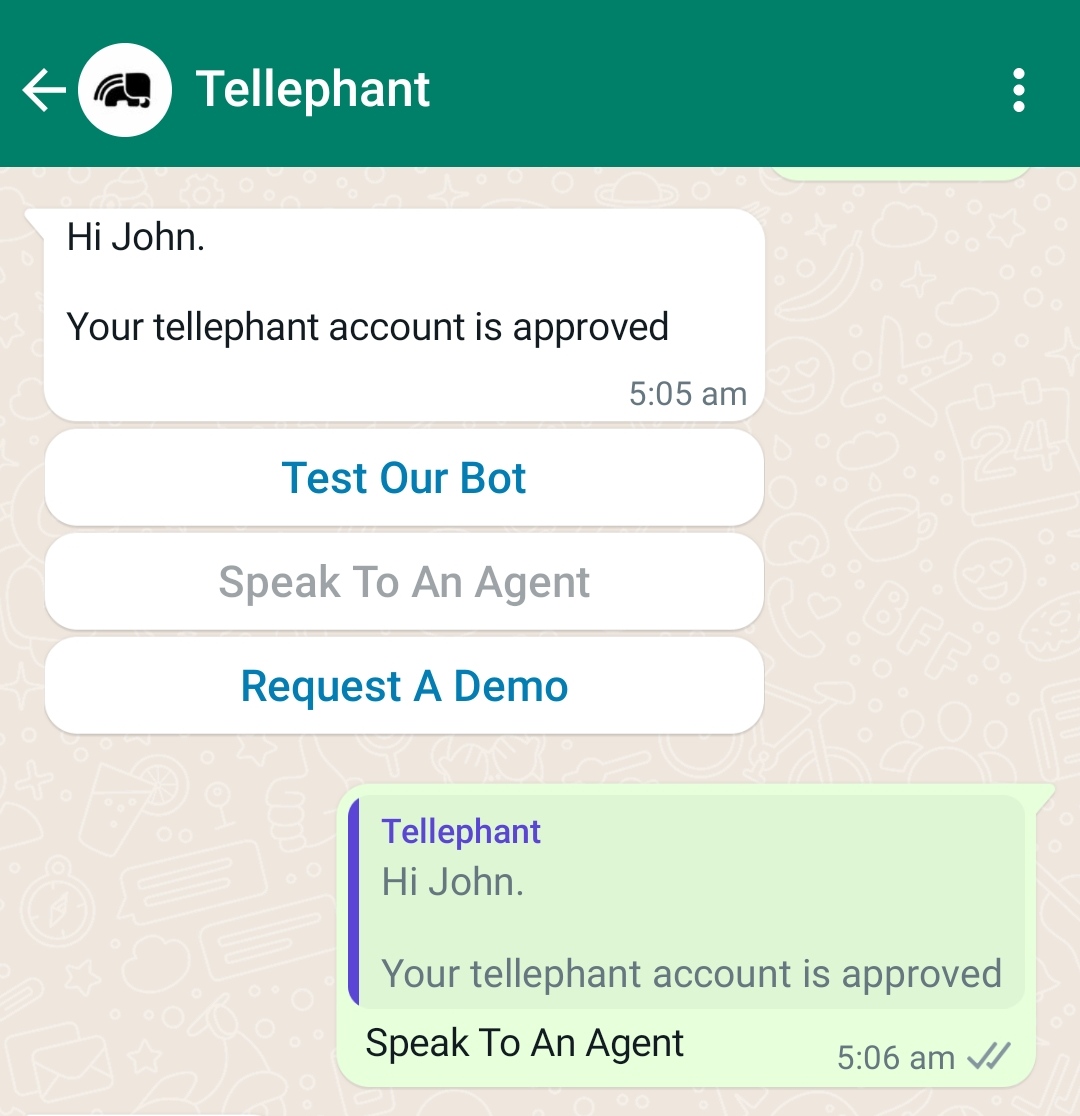
Template (Image with Quick Reply buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Image header |
url |
media | String | required | The url of the location where the image is stored |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
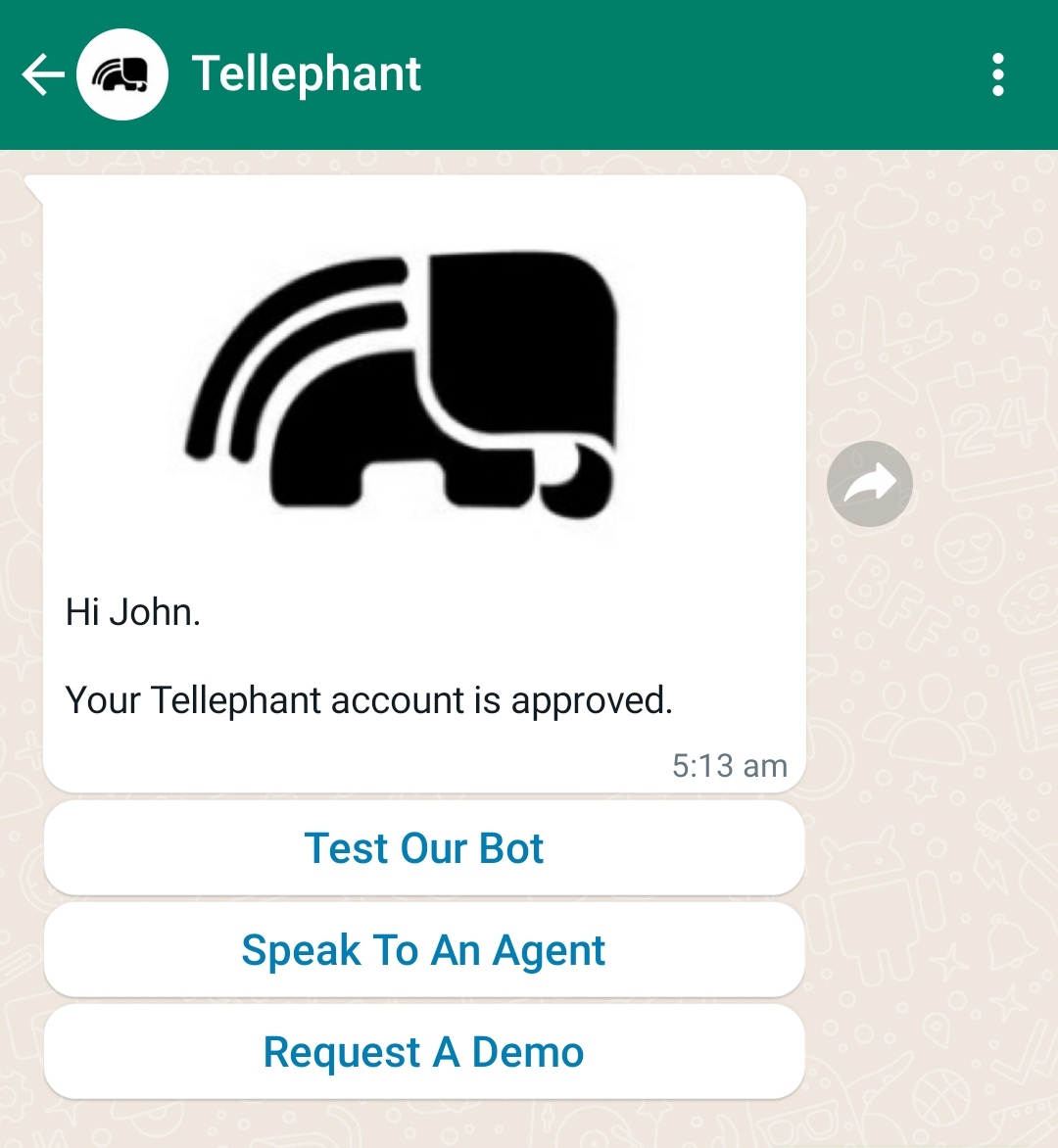
Template - Image with Static TEXT and Quick Reply buttons (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Image header |
url |
media | String | required | The url of the location where the image is stored |
Template (Document with Quick Reply buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Document header |
url |
media | String | required | The url of the location where the document is stored |
filename |
media | String | required | Document Name |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
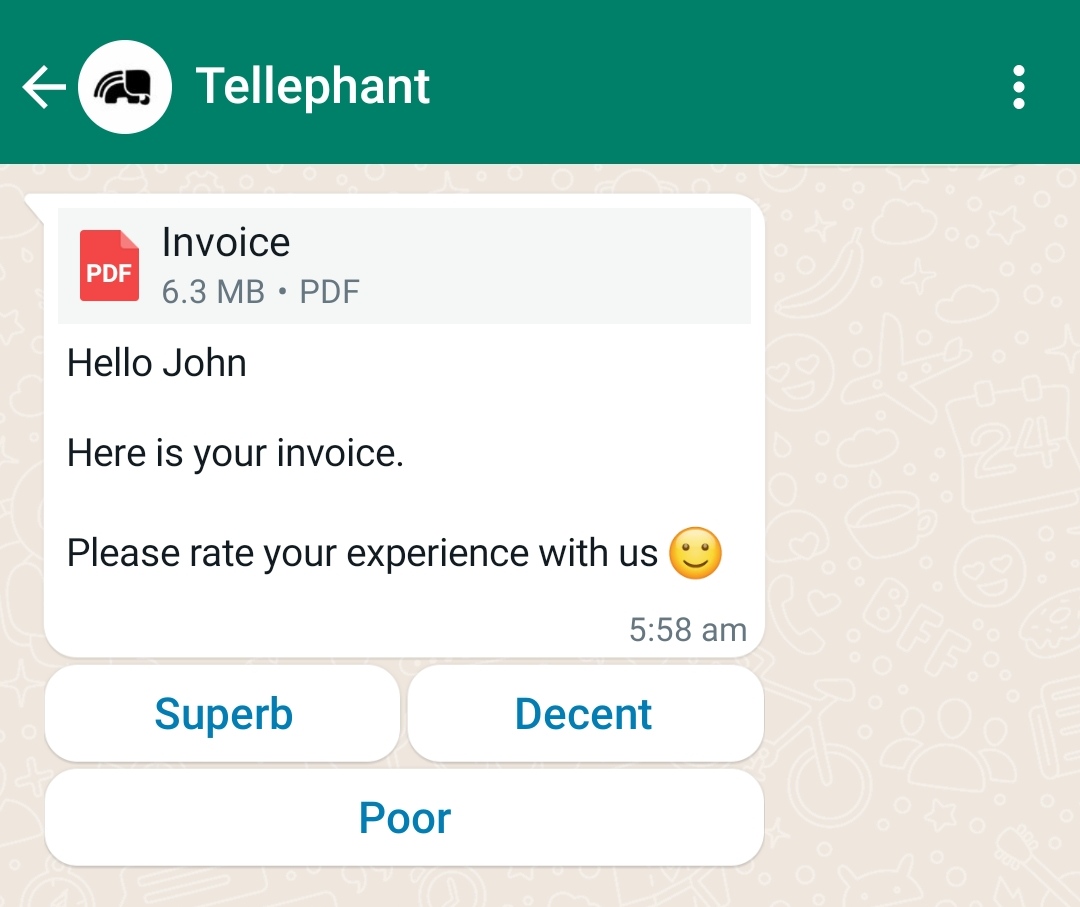
Template - Document with Static TEXT and Quick Reply buttons (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Document header |
url |
media | String | required | The url of the location where the document is stored |
filename |
media | String | required | Document Name |
Template (VIDEO with Quick Reply buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Video header |
url |
media | String | required | The url of the location where the video is stored |
caption |
media | String | optional | Additional caption for the video. |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
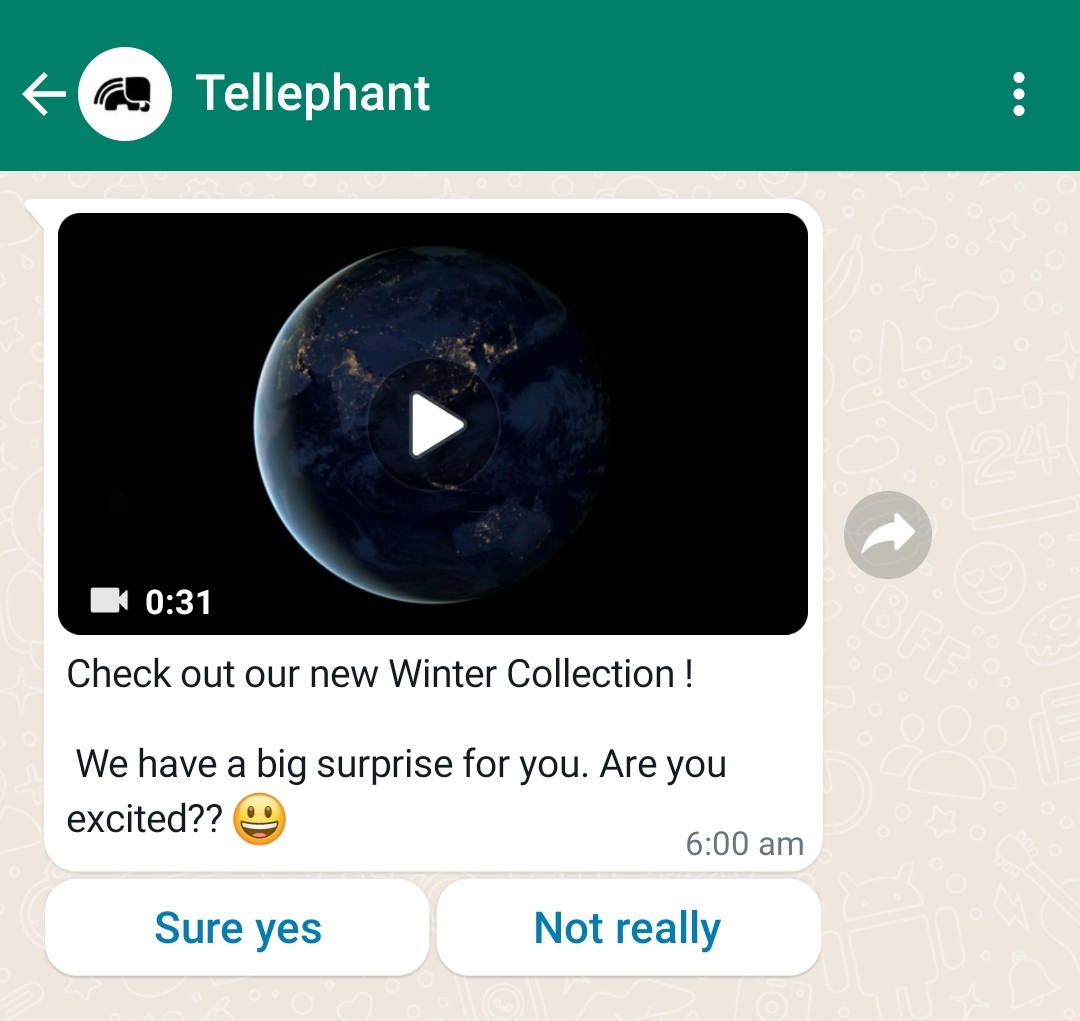
Template - VIDEO with Static Text and Quick Reply buttons (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Video header |
url |
media | String | required | The url of the location where the video is stored |
caption |
media | String | optional | Additional caption for the video. |
Template (LOCATION with Quick Reply buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Location component for WhatsApp Template |
latitude |
location | number(double) | required | Latitude component of location |
longitude |
location | number(double) | required | Longitude component of location |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
Template (CTA Buttons)
Template Messages Example request:
curl -X POST \
"https://api.tellephant.com/v1/send-message" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","to":919999999999,"channels":["whatsapp"],"whatsapp":{"contentType":"template","template":{"templateId":"dummy_template_name","language":"en","components":[]}}}'
const url = new URL(
"https://api.tellephant.com/v1/send-message"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
}
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/send-message',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'ABCDEFGHIJKLMNOPQRSTUVWXYZ',
'to' => 919999999999,
'channels' => [
'whatsapp',
],
'whatsapp' => [
'contentType' => 'template',
'template' => [
'templateId' => 'dummy_template_name',
'language' => 'en',
'components' => [],
],
],
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/send-message'
payload = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example Component Template (TEXT with CTA buttons):
"components" : [
{
"type" : "body",
"parameters" : [
{
"type" : "text",
"text" : "Dummy Text 1"
},
{
"type" : "text",
"text" : "Dummy Text 2"
}
]
}
]
Example Component Template - Static TEXT with CTA buttons (without any variables in text):
"components" : []
1Example Component Template (IMAGE with CTA buttons):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - IMAGE with Static TEXT and CTA buttons (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
}
]
Example Component Template (DOCUMENT with CTA buttons):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - DOCUMENT with Static TEXT and CTA buttons (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
}
]
Example Component Template (VIDEO with CTA buttons):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
}
]
Example Component Template - VIDEO with Static TEXT and CTA buttons (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
}
]
Example Component Template (Location with CTA buttons):
"components": [
{
"type": "header",
"parameters": [
{
"type": "location",
"location": {
"latitude": 51.5005765,
"longitude": 7.4954884
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Your text here"
}
]
}
]
Example response (200):
{
"success": true,
"messageId": "CAGHDVFHVFUDUYRFGUFJYR"
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (401):
{
"status": false,
"error": "Please subscribe to addon first"
}
Example response (403):
{
"status": false,
"error": "Balance Low! Please recharge!"
}
Send messages via this path.
POST v1/send-message
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
to |
integer | required | Number to send the message |
channels |
array | required | Channel(s) to be used. |
whatsapp.contentType |
String | required | Content type used |
whatsapp.template |
array | required | Template Details |
whatsapp.template.templateId |
String | required | Template identifier string |
whatsapp.template.language |
String | required | Language in the template |
whatsapp.template.components |
array | required | Template Data |
Note-
A Call to Action can be either an URL or phone number. For example, a direct link to your website/app, or the phone number to call your business.
URLs can be either static or have a dynamic parameter at the end of the URL.
No extra component is required in the request to send a Template with static CTA buttons. Example request for dynamic can be found in the next section.
Template (Text with CTA buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
body | array | required | Parameters to be used in Component |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
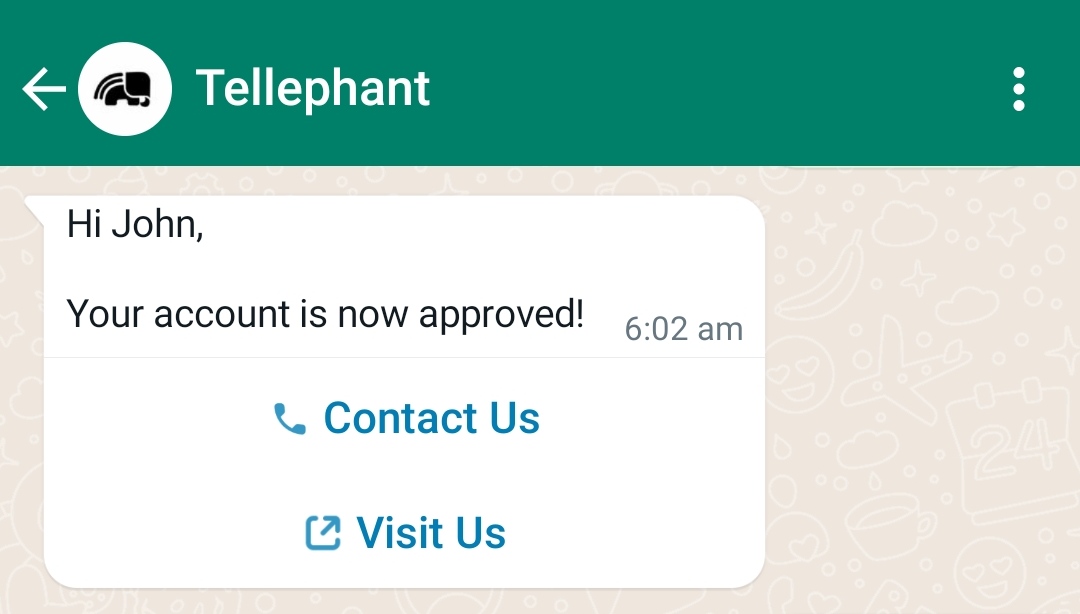
Template (Image with CTA buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Image header |
url |
media | String | required | The url of the location where the image is stored |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
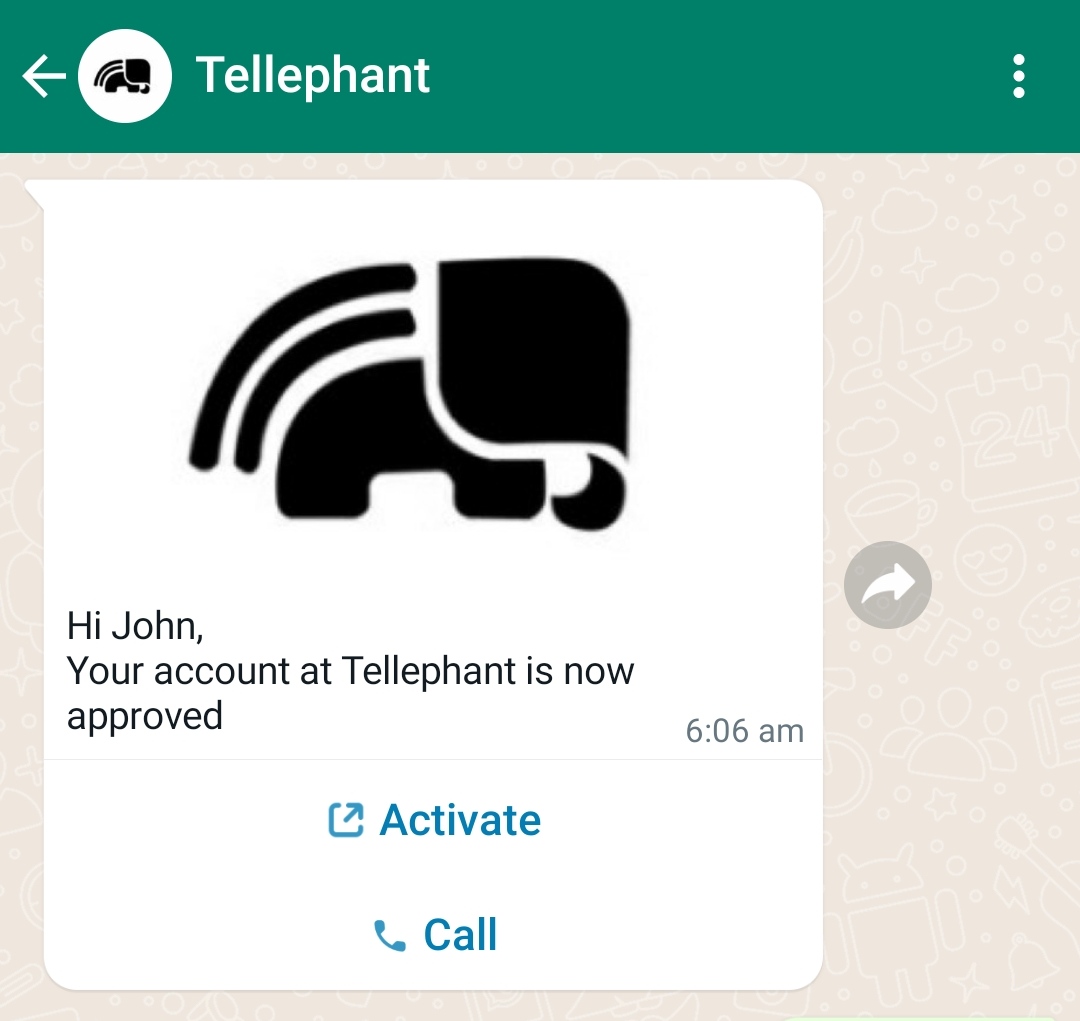
Template (Document with CTA buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Document header |
url |
media | String | required | The url of the location where the document is stored |
filename |
media | String | required | Document Name |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
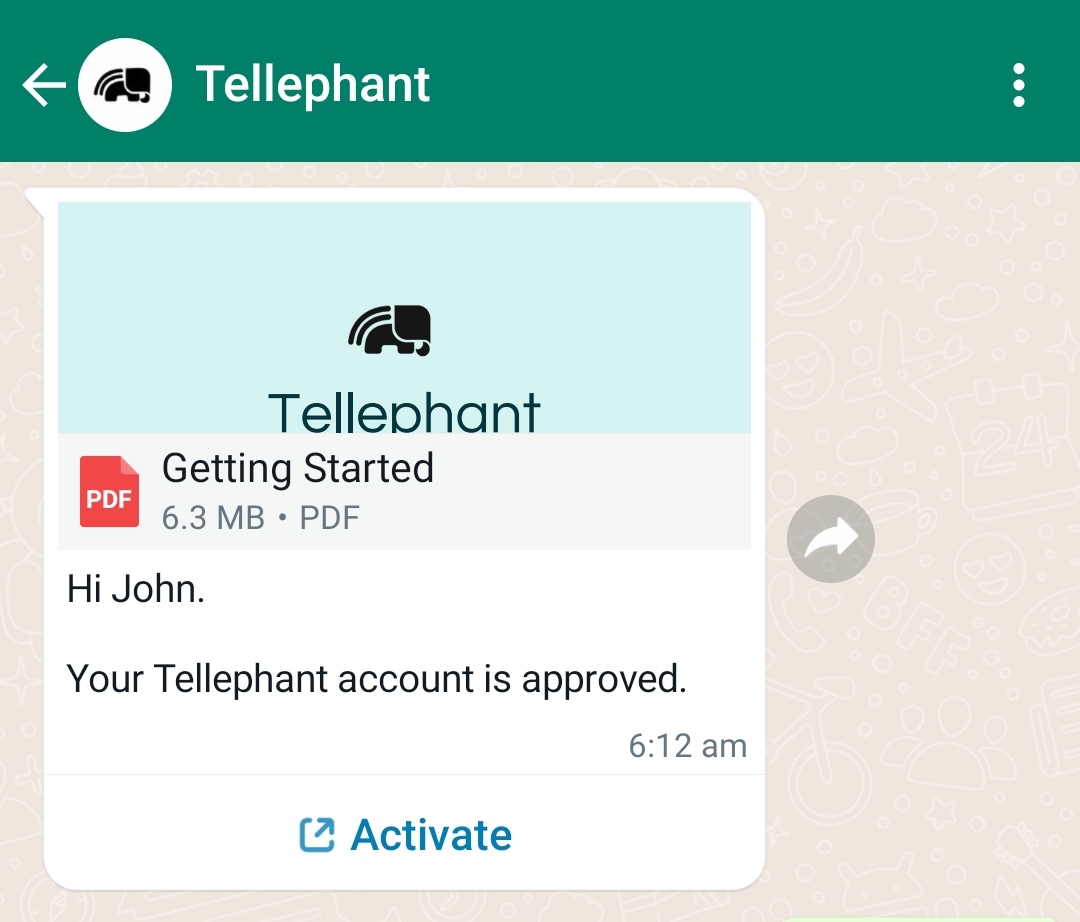
Template (VIDEO with CTA buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Media component for WhatsApp Template |
type |
media | String | required | Video header |
url |
media | String | required | The url of the location where the video is stored |
caption |
media | String | optional | Additional caption for the video. |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
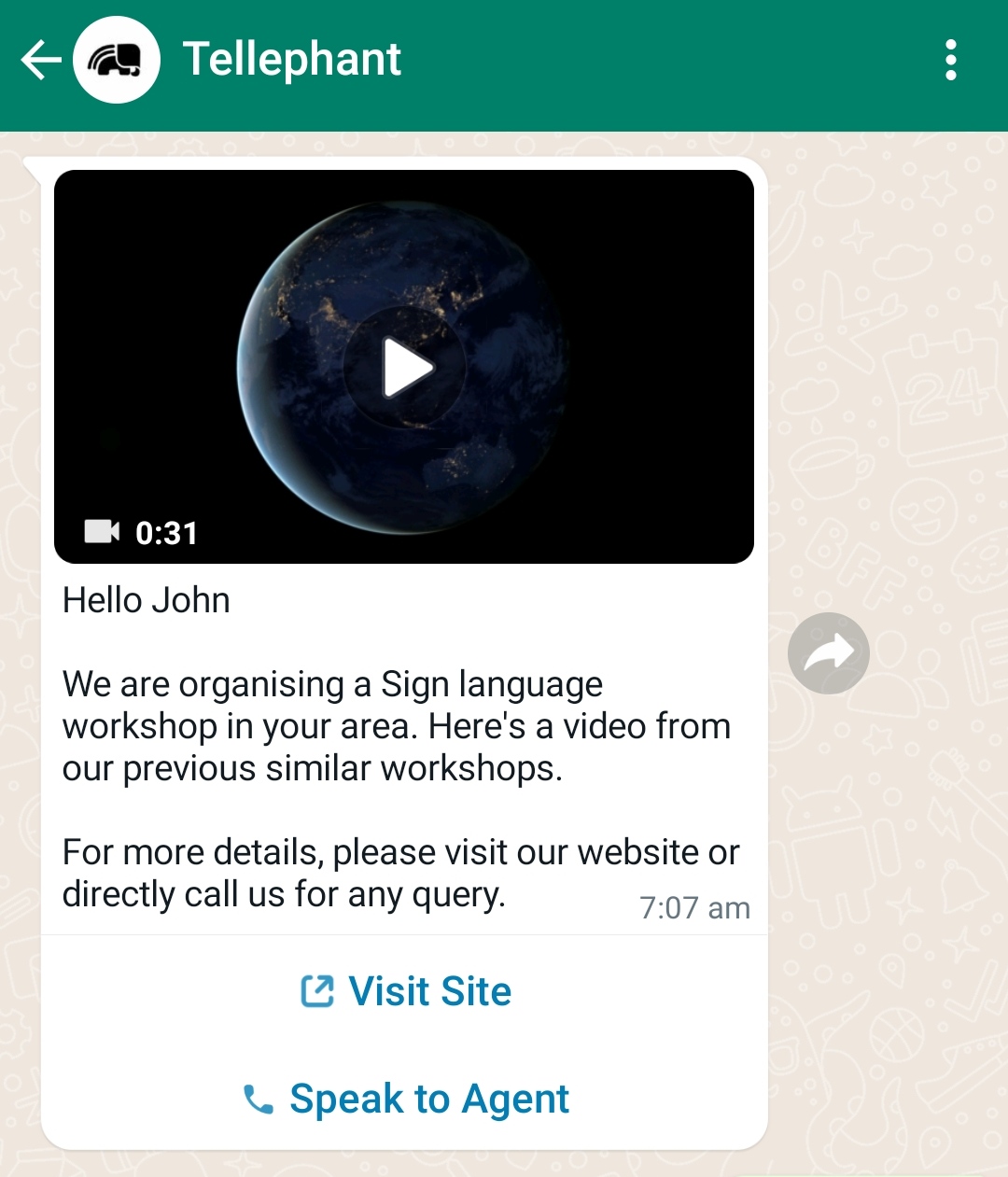
Template (LOCATION with CTA buttons)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters |
header | array | required | Parameters to be used in Component |
type |
header | String | required | Location component for WhatsApp Template |
latitude |
location | number(double) | required | Latitude component of location |
longitude |
location | number(double) | required | Longitude component of location |
type |
body | String | required | Text component for WhatsApp Template |
text |
body | String | required | The text to be sent |
Template (Dynamic CTA redirect Button)
Template Messages Example request:
curl -X POST \
"https://api.tellephant.com/v1/send-message" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","to":919999999999,"channels":["whatsapp"],"whatsapp":{"contentType":"template","template":{"templateId":"dummy_template_name","language":"en","components":[]}}}'
const url = new URL(
"https://api.tellephant.com/v1/send-message"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
}
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/send-message',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'ABCDEFGHIJKLMNOPQRSTUVWXYZ',
'to' => 919999999999,
'channels' => [
'whatsapp',
],
'whatsapp' => [
'contentType' => 'template',
'template' => [
'templateId' => 'dummy_template_name',
'language' => 'en',
'components' => [],
],
],
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/send-message'
payload = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example Component Template (TEXT with Dynamic CTA redirect button):
"components" : [
{
"type" : "body",
"parameters" : [
{
"type" : "text",
"text" : "Dummy Text 1"
},
{
"type" : "text",
"text" : "Dummy Text 2"
}
]
},
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
Example Component Template - Static TEXT with Dynamic CTA redirect button (without any variables in text):
"components" : [
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
1Example Component Template (IMAGE with Dynamic CTA redirect button):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
},
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
Example Component Template - IMAGE with Static TEXT and Dynamic CTA redirect button (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
},
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
Example Component Template (DOCUMENT with Dynamic CTA redirect button):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
},
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
Example Component Template - DOCUMENT with Static TEXT and Dynamic CTA redirect button (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
},
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
Example Component Template (VIDEO with Dynamic CTA redirect button):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
},
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
Example Component Template - VIDEO with Static TEXT and Dynamic CTA redirect button (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
},
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
Example Component Template (Location with Dynamic CTA redirect button):
"components": [
{
"type": "header",
"parameters": [
{
"type": "location",
"location": {
"latitude": 51.5005765,
"longitude": 7.4954884
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Your text here"
}
]
},
{
"type": "button",
"subType": "url",
"index": 0,
"parameters": [
{
"type": "text",
"text": "url_variable"
}
]
}
]
Example response (200):
{
"success": true,
"messageId": "CAGHDVFHVFUDUYRFGUFJYR"
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (401):
{
"status": false,
"error": "Please subscribe to addon first"
}
Example response (403):
{
"status": false,
"error": "Balance Low! Please recharge!"
}
Send messages via this path.
POST v1/send-message
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
to |
integer | required | Number to send the message |
channels |
array | required | Channel(s) to be used. |
whatsapp.contentType |
String | required | Content type used |
whatsapp.template |
array | required | Template Details |
whatsapp.template.templateId |
String | required | Template identifier string |
whatsapp.template.language |
String | required | Language in the template |
whatsapp.template.components |
array | required | Template Data |
Note-
URLs can be either static or have a dynamic parameter at the end of the URL.
Dynamic URLs can have one variable at the end of the URL, e.g. https://www.tellephant.com/my-url-{{1}}
Template (Text with Dynamic CTA redirect button)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
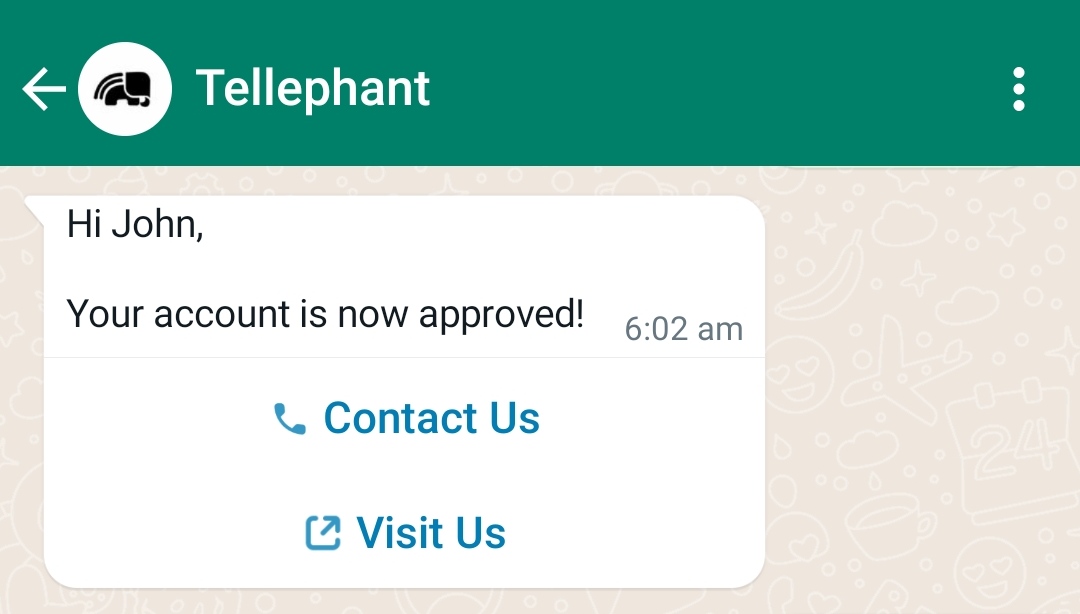
Template - Static Text with Dynamic CTA redirect button (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (Image with Dynamic CTA redirect button)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters.type |
header | String | required | Media component for WhatsApp Template |
media.type |
header | String | required | Image header |
media.url |
header | String | required | The url of the location where the image is stored |
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
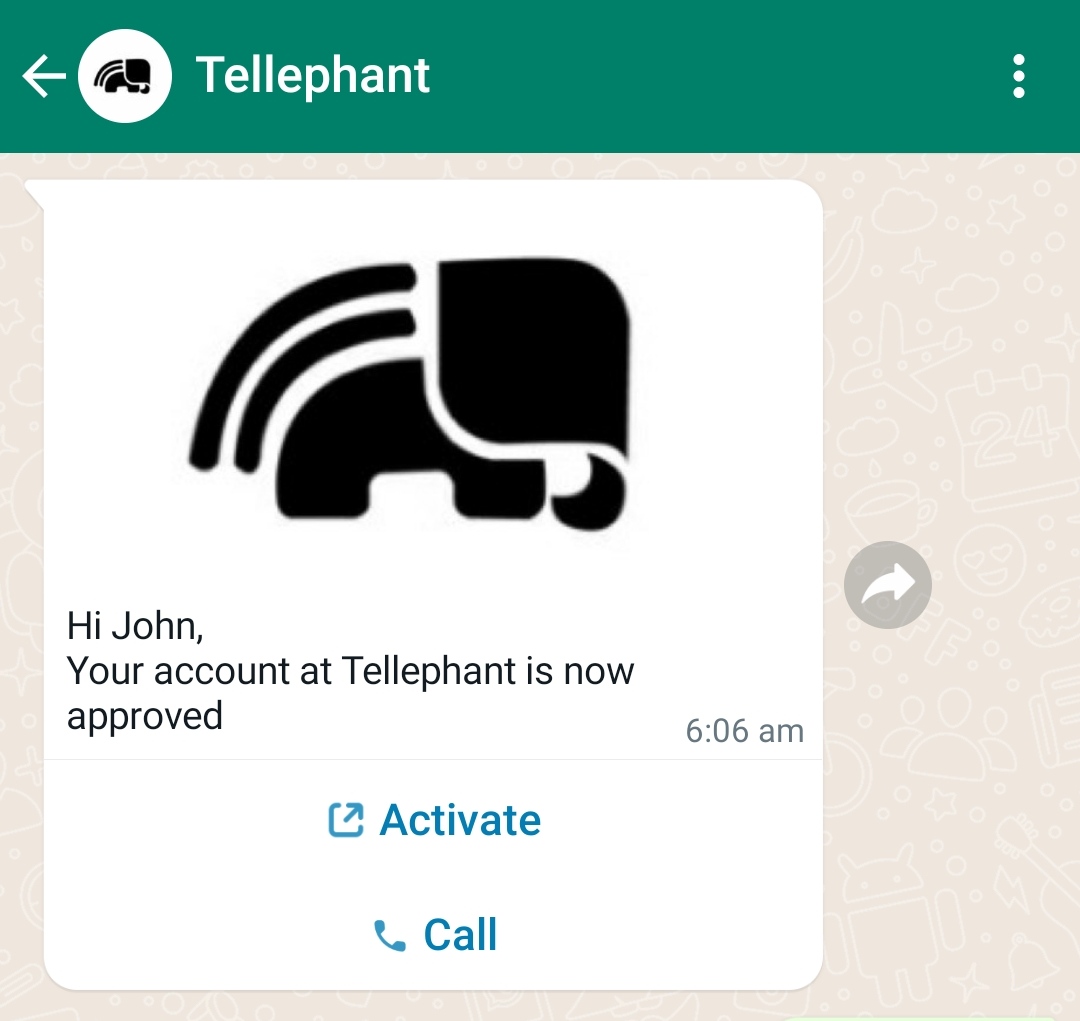
Template - Image with Static TEXT and Dynamic CTA redirect button (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters.type |
header | String | required | Media component for WhatsApp Template |
media.type |
header | String | required | Image header |
media.url |
header | String | required | The url of the location where the image is stored |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (Document with Dynamic CTA redirect button)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
paramters.type |
header | String | required | Document header |
media.url |
header | String | required | The url of the location where the document is stored |
media.filename |
header | String | required | Document Name |
media.type |
body | String | required | Text component for WhatsApp Template |
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
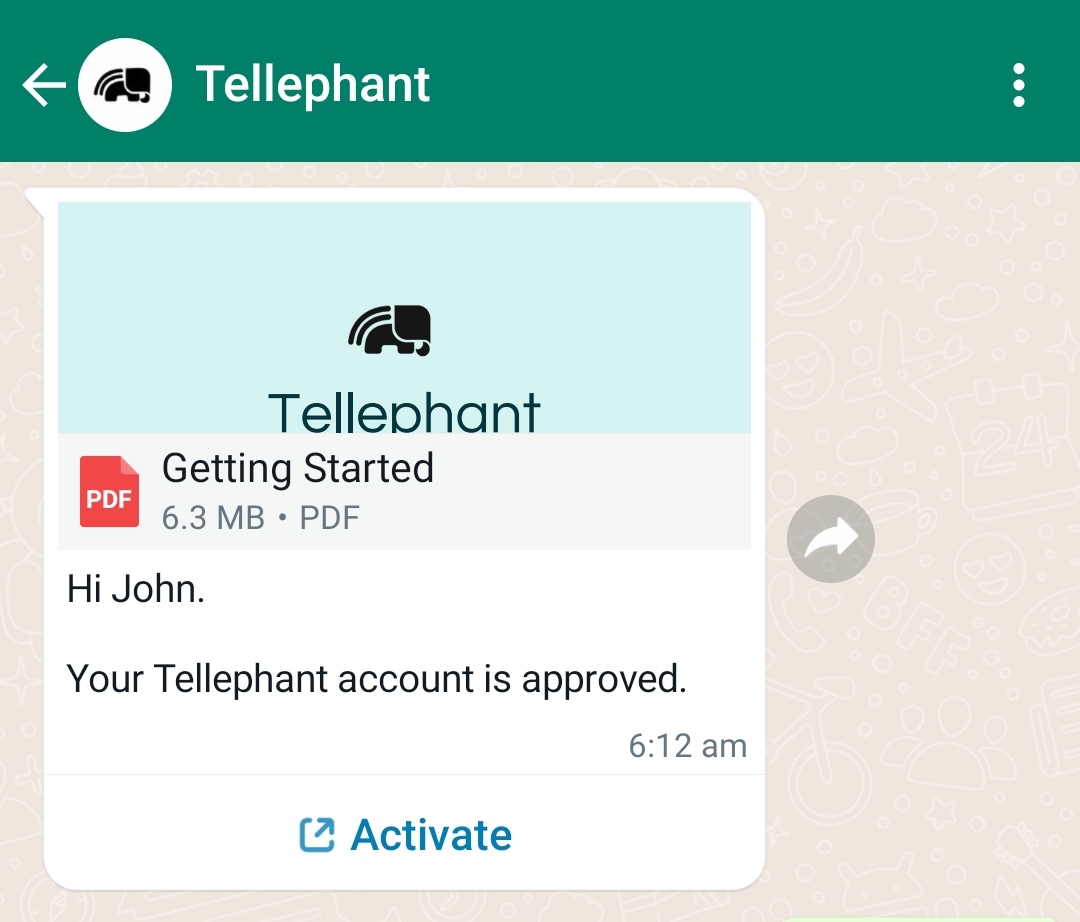
Template - Document with Static TEXT and Dynamic CTA redirect button (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
paramters.type |
header | String | required | Document header |
media.url |
header | String | required | The url of the location where the document is stored |
media.filename |
header | String | required | Document Name |
media.type |
header | String | required | Text component for WhatsApp Template |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (VIDEO with Dynamic CTA redirect button)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
paramters.type |
header | String | required | Video header |
media.type |
header | String | required | Video header |
media.url |
header | String | required | The url of the location where the video is stored |
media.caption |
header | String | optional | Additional caption for the video. |
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
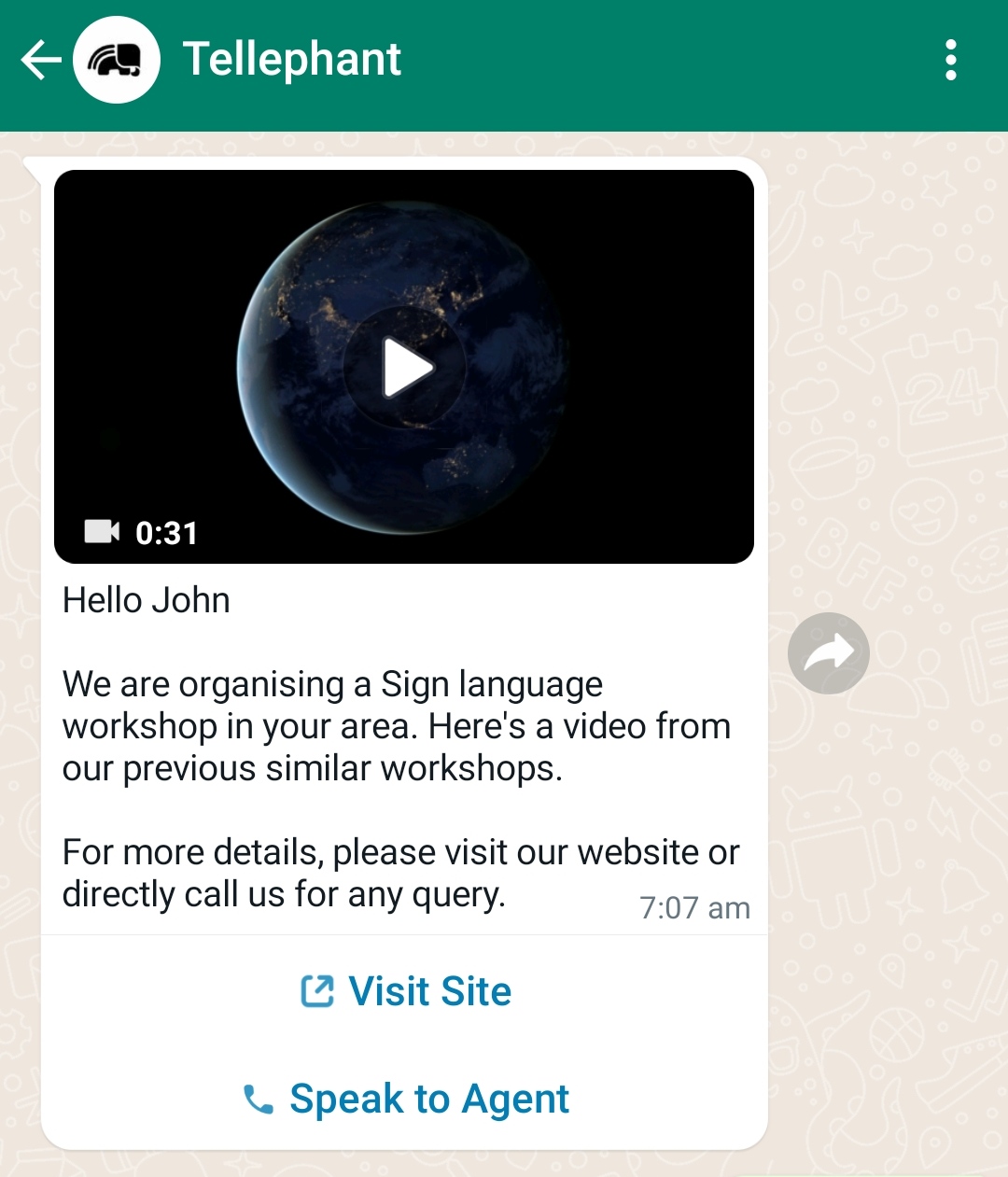
Template - VIDEO with Static TEXT and Dynamic CTA redirect button (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
paramters.type |
header | String | required | Video header |
media.type |
header | String | required | Video header |
media.url |
header | String | required | The url of the location where the video is stored |
media.caption |
header | String | optional | Additional caption for the video. |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (LOCATION with Dynamic CTA redirect button)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters.type |
header | String | required | Location component for WhatsApp Template |
location.latitude |
header | number(double) | required | Latitude component of location |
location.longitude |
header | number(double) | required | Longitude component of location |
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (Click Tracking)
Template Messages Example request:
curl -X POST \
"https://api.tellephant.com/v1/send-message" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","to":919999999999,"channels":["whatsapp"],"whatsapp":{"contentType":"template","template":{"templateId":"dummy_template_name","language":"en","components":[]}}, "destination_url":"https://app.tellephant.com"}'
const url = new URL(
"https://api.tellephant.com/v1/send-message"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
},
"destination_url" : "https://app.tellephant.com"
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/send-message',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'ABCDEFGHIJKLMNOPQRSTUVWXYZ',
'to' => 919999999999,
'channels' => [
'whatsapp',
],
'whatsapp' => [
'contentType' => 'template',
'template' => [
'templateId' => 'dummy_template_name',
'language' => 'en',
'components' => [],
],
],
'destination_url' => 'https://app.tellephant.com'
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/send-message'
payload = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {
"contentType": "template",
"template": {
"templateId": "dummy_template_name",
"language": "en",
"components": []
}
},
"destination_url" : "https://app.tellephant.com"
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example Component Template (TEXT with Click Tracking URL):
"components" : [
{
"type" : "body",
"parameters" : [
{
"type" : "text",
"text" : "Dummy Text 1"
},
{
"type" : "text",
"text" : "Dummy Text 2"
}
]
},
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
Example Component Template - Static TEXT with Click Tracking URL (without any variables in text):
"components" : [
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
1Example Component Template (IMAGE with Click Tracking URL):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
},
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
Example Component Template - IMAGE with Static TEXT and Click Tracking URL (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
}
]
},
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
Example Component Template (DOCUMENT with Click Tracking URL):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
},
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
Example Component Template - DOCUMENT with Static TEXT and Click Tracking URL (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename": "Sample PDF"
}
}
]
},
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
Example Component Template (VIDEO with Click Tracking URL):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Dummy Text 1"
},
{
"type": "text",
"text": "Dummy Text 2"
}
]
},
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
Example Component Template - VIDEO with Static TEXT and Click Tracking URL (without any variables in text):
"components": [
{
"type": "header",
"parameters": [
{
"type": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
}
]
},
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
Example Component Template (Location with Click Tracking URL):
"components": [
{
"type": "header",
"parameters": [
{
"type": "location",
"location": {
"latitude": 51.5005765,
"longitude": 7.4954884
}
}
]
},
{
"type": "body",
"parameters": [
{
"type": "text",
"text": "Your text here"
}
]
},
{
"type": "button",
"subType": "url",
"parameters": [
{
"type": "text"
}
]
}
]
Example response (200):
{
"success": true,
"messageId": "CAGHDVFHVFUDUYRFGUFJYR"
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (401):
{
"status": false,
"error": "Please subscribe to addon first"
}
Example response (403):
{
"status": false,
"error": "Balance Low! Please recharge!"
}
Send messages via this path.
POST v1/send-message
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
to |
integer | required | Number to send the message |
channels |
array | required | Channel(s) to be used. |
whatsapp.contentType |
String | required | Content type used |
whatsapp.template |
array | required | Template Details |
whatsapp.template.templateId |
String | required | Template identifier string |
whatsapp.template.language |
String | required | Language in the template |
whatsapp.template.components |
array | required | Template Data |
destination_url |
String | required | Destination URL for redirection |
Check out our new Click Tracking Templates!
Template (Text with Click Tracking URL)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
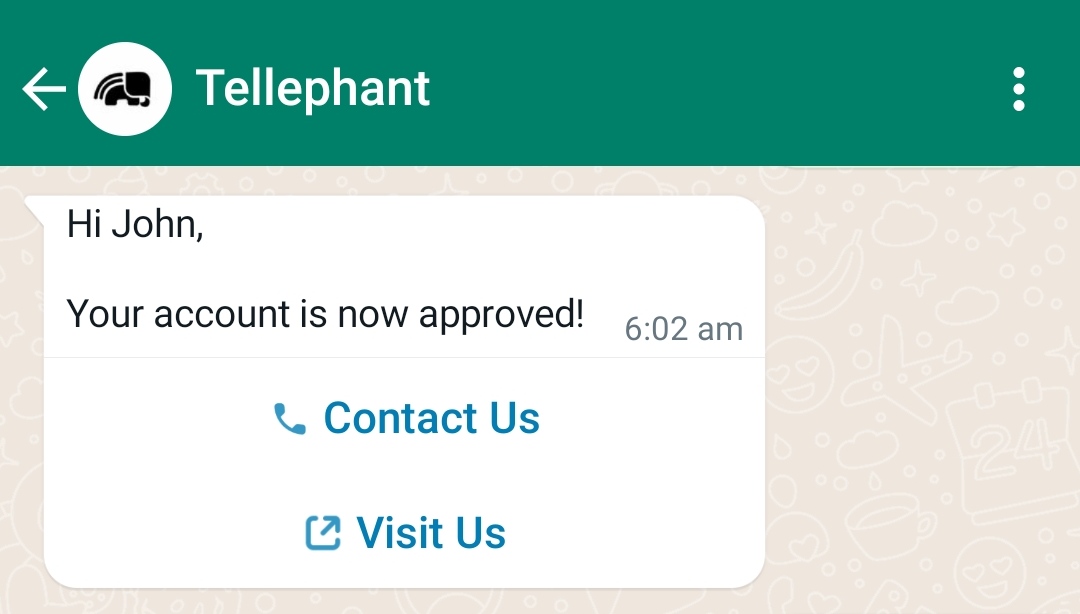
Template - Static Text with Click Tracking URL (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (Image with Click Tracking URL)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters.type |
header | String | required | Media component for WhatsApp Template |
media.type |
header | String | required | Image header |
media.url |
header | String | required | The url of the location where the image is stored |
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
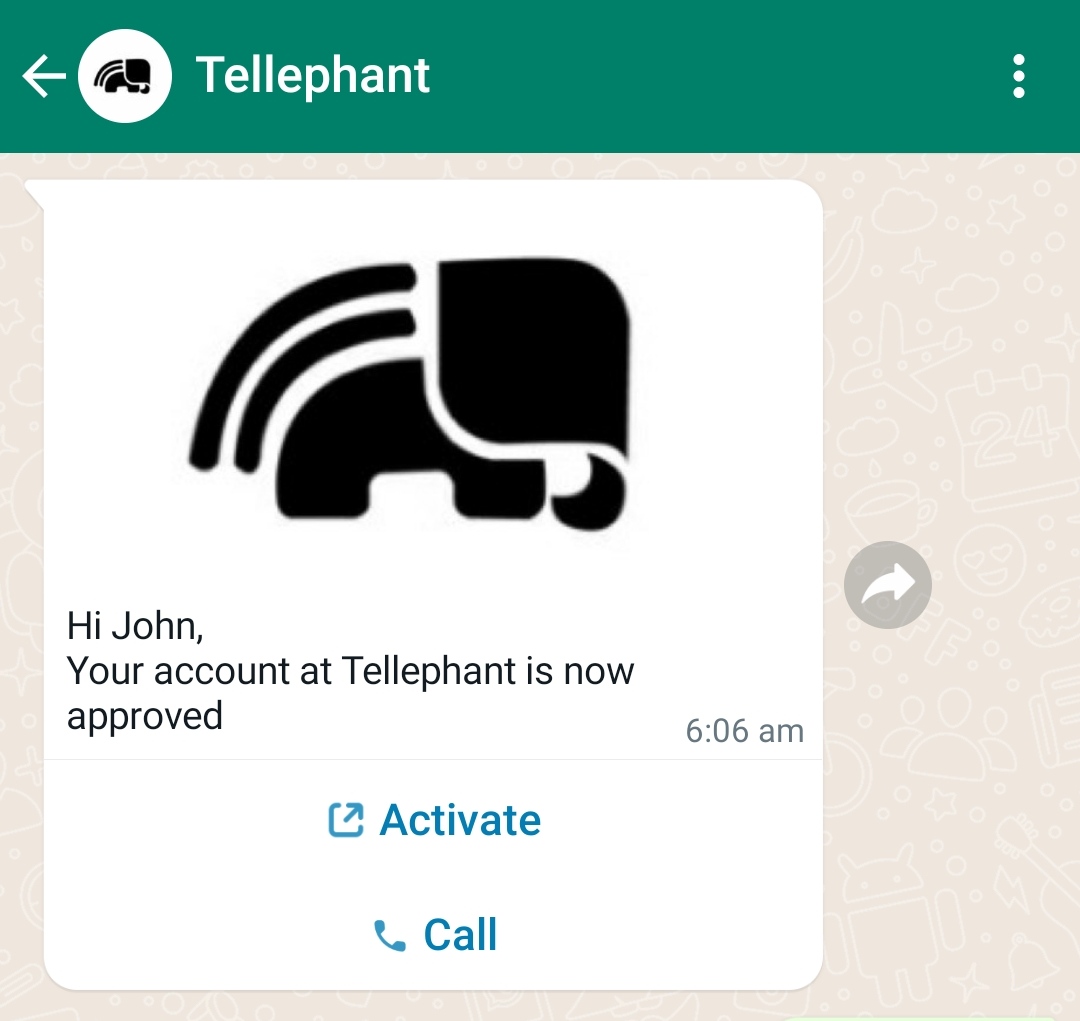
Template - Image with Static TEXT and Click Tracking URL (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters.type |
header | String | required | Media component for WhatsApp Template |
media.type |
header | String | required | Image header |
media.url |
header | String | required | The url of the location where the image is stored |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (Document with Click Tracking URL)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
paramters.type |
header | String | required | Document header |
media.url |
header | String | required | The url of the location where the document is stored |
media.filename |
header | String | required | Document Name |
media.type |
body | String | required | Text component for WhatsApp Template |
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
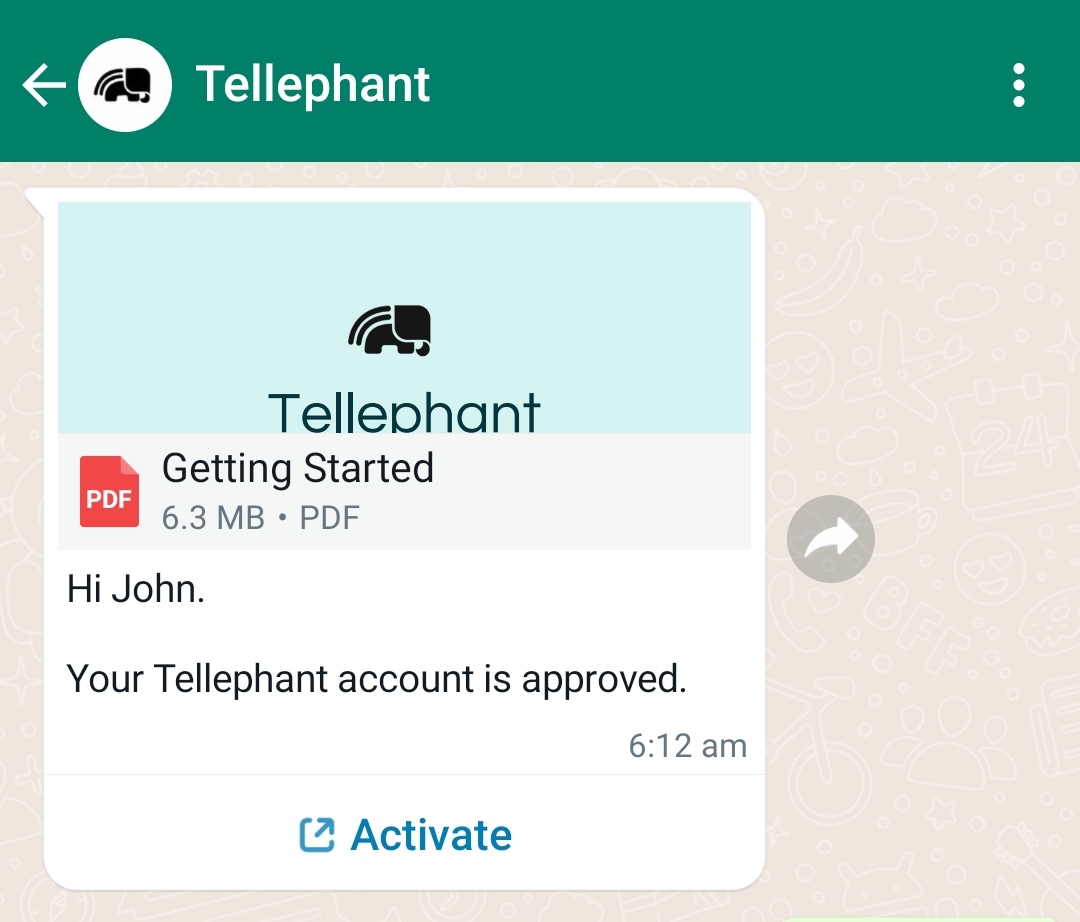
Template - Document with Static TEXT and Click Tracking URL (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
paramters.type |
header | String | required | Document header |
media.url |
header | String | required | The url of the location where the document is stored |
media.filename |
header | String | required | Document Name |
media.type |
header | String | required | Text component for WhatsApp Template |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (VIDEO with Click Tracking URL)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
paramters.type |
header | String | required | Video header |
media.type |
header | String | required | Video header |
media.url |
header | String | required | The url of the location where the video is stored |
media.caption |
header | String | optional | Additional caption for the video. |
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
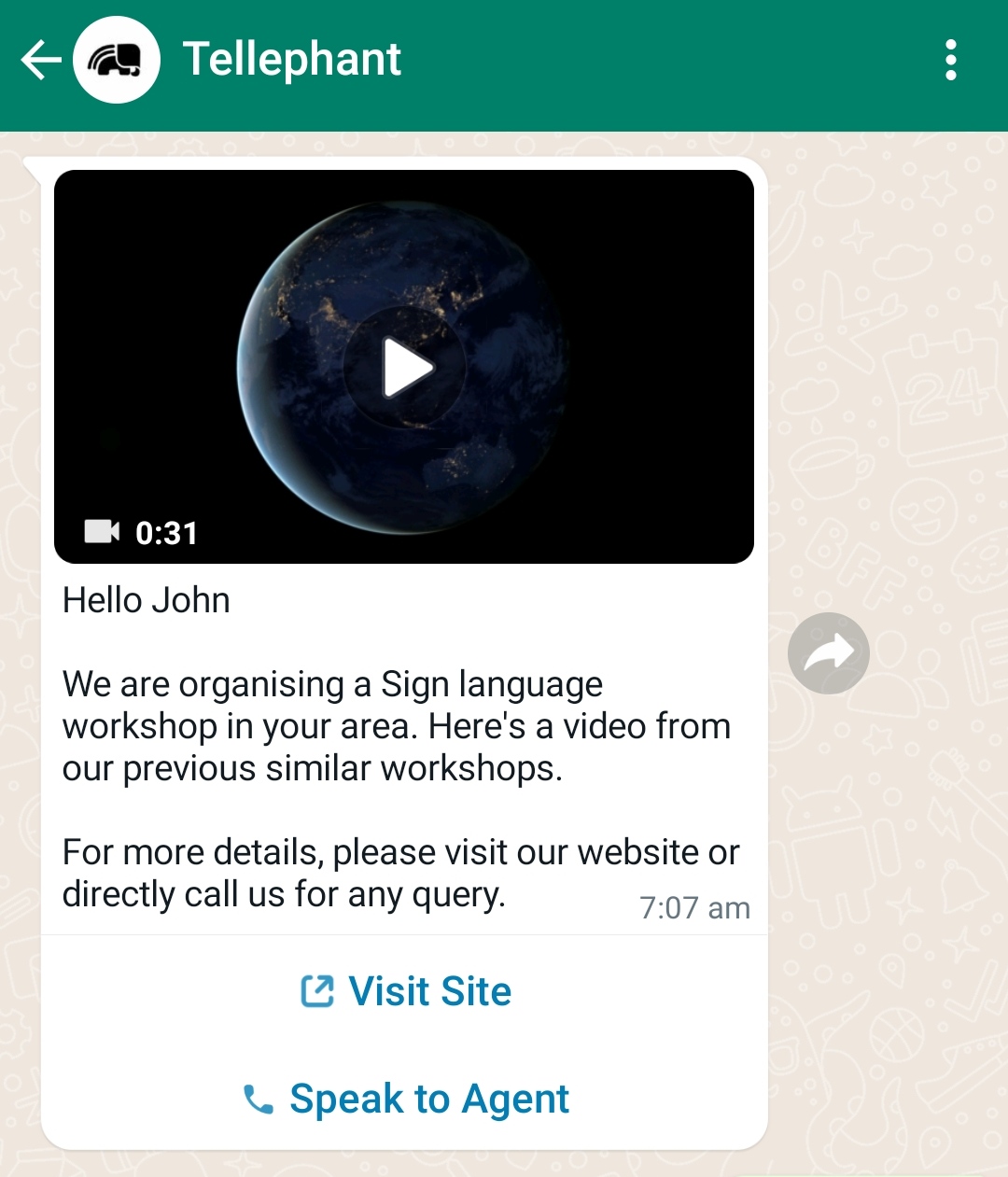
Template - VIDEO with Static TEXT and Click Tracking URL (without any variables in text)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
paramters.type |
header | String | required | Video header |
media.type |
header | String | required | Video header |
media.url |
header | String | required | The url of the location where the video is stored |
media.caption |
header | String | optional | Additional caption for the video. |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Template (LOCATION with Click Tracking URL)
Parameter | In | Type | Status | Description |
---|---|---|---|---|
parameters.type |
header | String | required | Location component for WhatsApp Template |
location.latitude |
header | number(double) | required | Latitude component of location |
location.longitude |
header | number(double) | required | Longitude component of location |
parameter.type |
body | String | required | Text component for WhatsApp Template |
parameter.text |
body | String | required | The text to be sent |
type |
button | String | required | CTA button component for WhatsApp Template |
subType |
button | String | required | Type of CTA button |
index |
button | numeric | required | 0 if URL button precedes PHONE button, and 1 otherwise |
parameter.type |
button | String | required | Data type of URL variable |
parameter.text |
button | String | required | Variable put in the dynamic URL |
Session Messages
Session Messages Example request:
curl -X POST \
"https://api.tellephant.com/v1/send-message" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","to":919999999999,"channels":["whatsapp"],"whatsapp": {}}'
const url = new URL(
"https://api.tellephant.com/v1/send-message"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {}
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/send-message',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'ABCDEFGHIJKLMNOPQRSTUVWXYZ',
'to' => 919999999999,
'channels' => [
'whatsapp',
],
'whatsapp' => [],
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/send-message'
payload = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"channels": [
"whatsapp"
],
"whatsapp": {}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example Component Session Message (TEXT):
"whatsapp" : {
"contentType" : "text",
"text" : "You message here"
}
Example Component Session Message (IMAGE):
"whatsapp": {
"contentType": "media",
"media": {
"type": "image",
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
"caption": "Some text description"
}
}
Example Component Session Message (DOCUMENT):
"whatsapp": {
"contentType": "media",
"media": {
"type": "document",
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename" : "Sample PDF"
}
}
Example Component Session Message (STICKER):
"whatsapp": {
"contentType": "media",
"media": {
"type": "sticker",
"url": "https://assets.tellephant.com/dummy-media/sample.webp"
}
}
Example Component Session Message (VIDEO):
"whatsapp": {
"contentType": "media",
"media": {
"type": "video",
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
"caption" : "Sample Video"
}
}
Example Component Session Message (AUDIO):
"whatsapp": {
"contentType": "media",
"media": {
"type": "audio",
"url": "https://assets.tellephant.com/dummy-media/audio.mp4",
"caption" : "Sample Audio"
}
}
Example Component Session Message (LOCATION):
"whatsapp": {
"contentType": "location",
"location": {
"longitude": -73.9855,
"latitude": 40.7580,
"name" : "Times Square",
"address" : "Manhattan, NY 10036, United States"
}
}
Example Component Session Message (CONTACT):
"whatsapp": {
"contentType": "contacts",
"contacts": [
{
"name": {
"formattedName": "XYZ ABC",
"firstName" : "XYZ"
},
"phones": [
{
"phone": "919999999999",
"type": "WORK"
}
]
}
]
}
Example Component Session Message (Interactive List):
"whatsapp": {
"contentType": "interactive",
"interactive": {
"subType": "list",
"components": {
"header": {
"type": "text",
"text": "Choose your menu"
},
"body": {
"type": "text",
"text": "Hi Sarah, select your menu from the bottom list. Dressings and toppings are selected later on"
},
"footer": {
"type": "text",
"text": "Your tellephant food team"
},
"list": {
"title": "Your menu",
"sections": [
{
"title": "Vegan",
"rows": [
{
"payload": "green-dream-34987-234897234-234",
"title": "Green dream",
"description": "A bowl full of tasty leaves, soy beans and cucumber"
},
{
"payload": "rainbow-meets-rice-34987-234897234-234",
"title": "Rainbow meets rice",
"description": "A colorful selection of vegetables on a cozy bed of basmati rice"
}
]
},
{
"title": "Vegetarian",
"rows": [
{
"payload": "italo-classic-34987-234897234-234",
"title": "Italo Classic",
"description": "Slices of tomates, with plucked pieces of mozzarella and basil leaves"
},
{
"payload": "egg-and-peas-34987-234897234-234",
"title": "Egg & Peas",
"description": "Tasty slices of eggs, on a whole wheat pasta salad with peas"
}
]
}
]
}
}
}
}
Example Component Session Message (Interactive Reply Buttons) - Text Header:
"whatsapp": {
"contentType": "interactive",
"interactive": {
"subType": "buttons",
"components": {
"header": {
"type": "text",
"text": "Your request is queued"
},
"body": {
"type": "text",
"text": "How would you rate your bot experience"
},
"footer": {
"type": "text",
"text": "Your service bot"
},
"buttons": [
{
"type": "reply",
"reply": {
"payload": "987298-40980jvkdm9-234234234",
"title": "Poor"
}
},
{
"type": "reply",
"reply": {
"payload": "987298-dsfgjlkhgdf-dg09u834334",
"title": "OK"
}
},
{
"type": "reply",
"reply": {
"payload": "9080923445nlkjß0_gß0923845083245dfg",
"title": "Good"
}
}
]
}
}
}
Example Component Session Message (Interactive Reply Buttons) - Image Header:
"whatsapp": {
"contentType": "interactive",
"interactive": {
"subType": "buttons",
"components": {
"header": {
"type": "image",
"image": {
"url": "https://assets.tellephant.com/dummy-media/sample.jpeg"
}
},
"body": {
"type": "text",
"text": "How would you rate your bot experience"
},
"footer": {
"type": "text",
"text": "Your service bot"
},
"buttons": [
{
"type": "reply",
"reply": {
"payload": "987298-40980jvkdm9-234234234",
"title": "Poor"
}
},
{
"type": "reply",
"reply": {
"payload": "987298-dsfgjlkhgdf-dg09u834334",
"title": "OK"
}
},
{
"type": "reply",
"reply": {
"payload": "9080923445nlkjß0_gß0923845083245dfg",
"title": "Good"
}
}
]
}
}
}
Example Component Session Message (Interactive Reply Buttons) - Document Header:
"whatsapp": {
"contentType": "interactive",
"interactive": {
"subType": "buttons",
"components": {
"header": {
"type": "document",
"document": {
"url": "https://assets.tellephant.com/dummy-media/sample.pdf",
"filename" : "Sample"
}
},
"body": {
"type": "text",
"text": "How would you rate your bot experience"
},
"footer": {
"type": "text",
"text": "Your service bot"
},
"buttons": [
{
"type": "reply",
"reply": {
"payload": "987298-40980jvkdm9-234234234",
"title": "Poor"
}
},
{
"type": "reply",
"reply": {
"payload": "987298-dsfgjlkhgdf-dg09u834334",
"title": "OK"
}
},
{
"type": "reply",
"reply": {
"payload": "9080923445nlkjß0_gß0923845083245dfg",
"title": "Good"
}
}
]
}
}
}
Example Component Session Message (Interactive Reply Buttons) - Video Header:
"whatsapp": {
"contentType": "interactive",
"interactive": {
"subType": "buttons",
"components": {
"header": {
"type": "video",
"video": {
"url": "https://assets.tellephant.com/dummy-media/sample.mp4"
}
},
"body": {
"type": "text",
"text": "How would you rate your bot experience"
},
"footer": {
"type": "text",
"text": "Your service bot"
},
"buttons": [
{
"type": "reply",
"reply": {
"payload": "987298-40980jvkdm9-234234234",
"title": "Poor"
}
},
{
"type": "reply",
"reply": {
"payload": "987298-dsfgjlkhgdf-dg09u834334",
"title": "OK"
}
},
{
"type": "reply",
"reply": {
"payload": "9080923445nlkjß0_gß0923845083245dfg",
"title": "Good"
}
}
]
}
}
}
Send session messages via this path.
POST v1/send-message
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
to |
integer | required | Number to send the otp |
channels |
array | required | Channel(s) to be used. |
whatsapp |
array | required | Session message type and other details. |
Session Message (Text)
Parameter | Type | Status | Description |
---|---|---|---|
contentType |
string | required | Text header for WhatsApp Session message |
text |
String | required | The text to be sent |
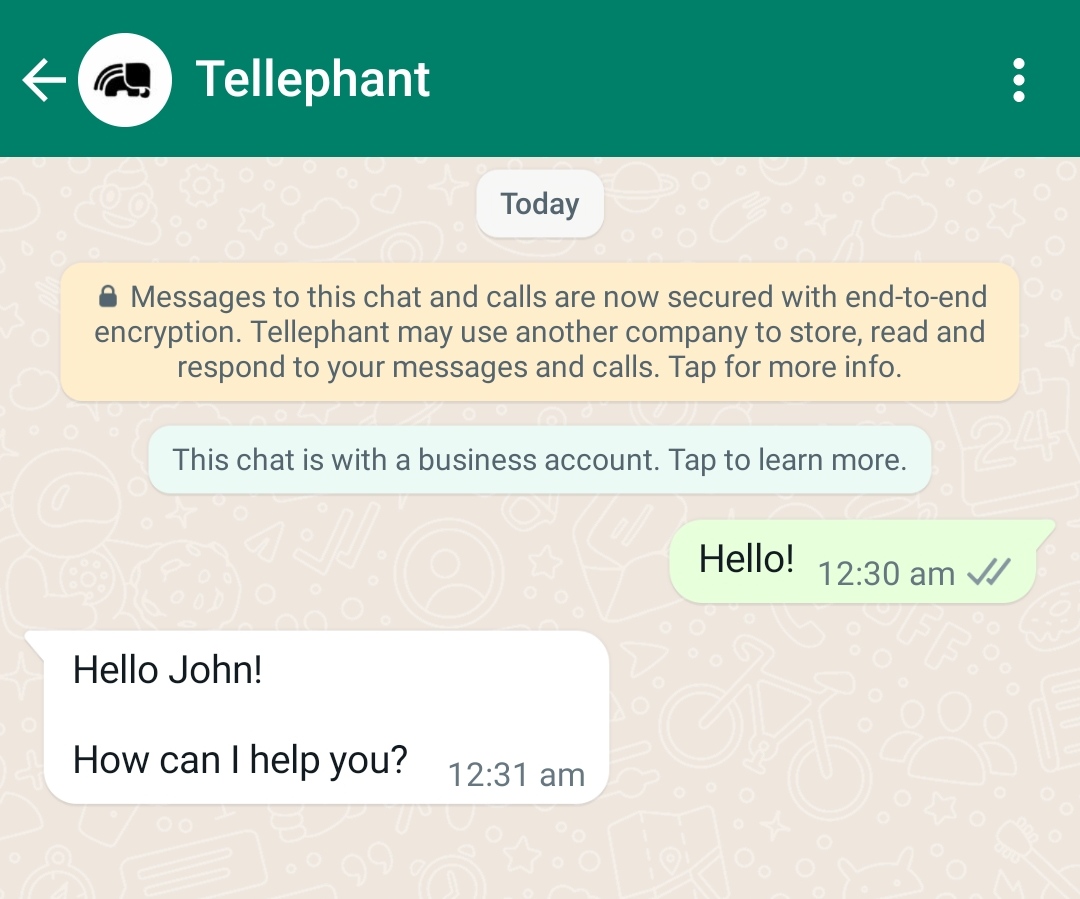
Session Message (IMAGE)
Parameter | Type | Status | Description |
---|---|---|---|
contentType |
string | required | Media header for WhatsApp Session message |
type |
String | required | Type of media used |
url |
String | required | Location where the image is stored |
caption |
String | required | Text description alongwith |
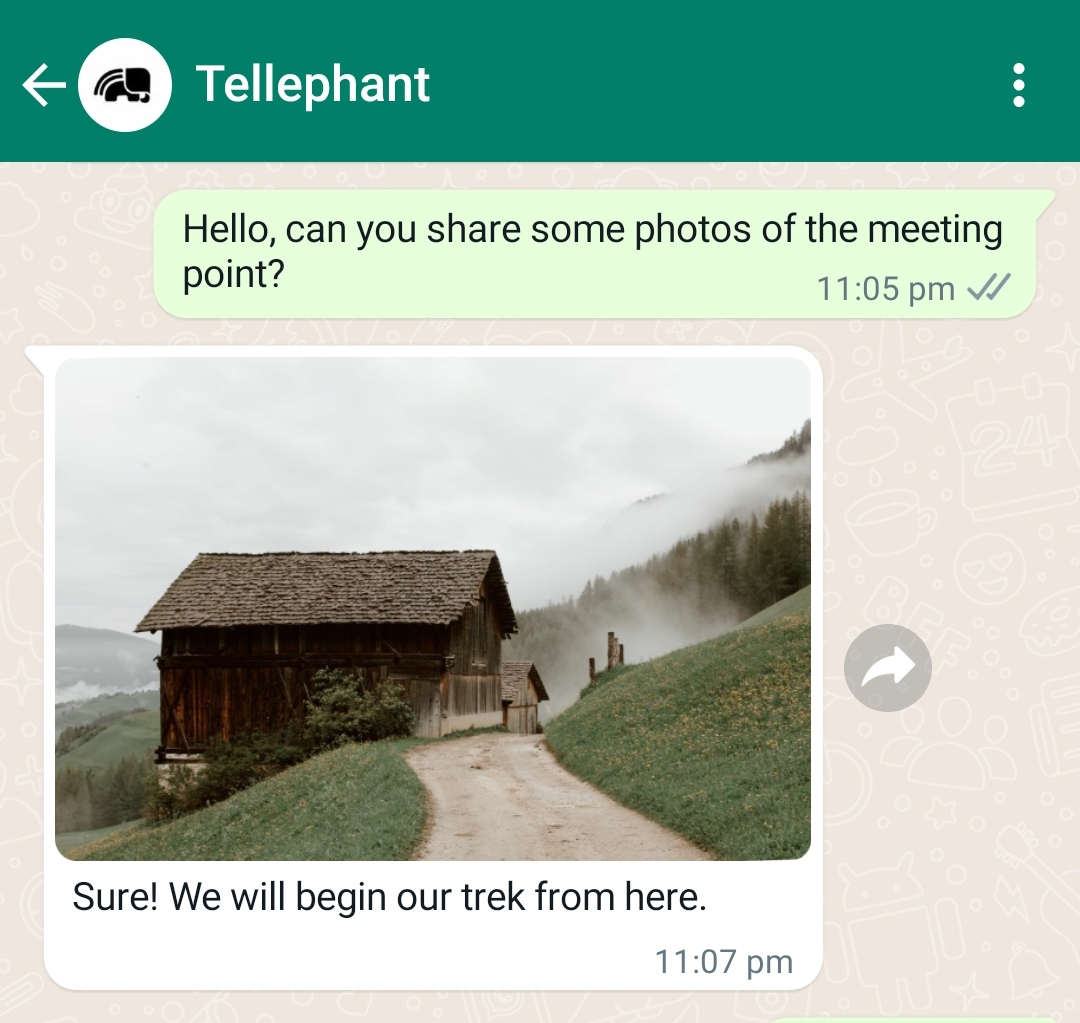
Session Message (DOCUMENT)
Parameter | Type | Status | Description |
---|---|---|---|
contentType |
string | required | Media header for WhatsApp Session message |
type |
String | required | Type of media used |
url |
String | required | Location where the document is stored |
filename |
String | Required | Title for the document |
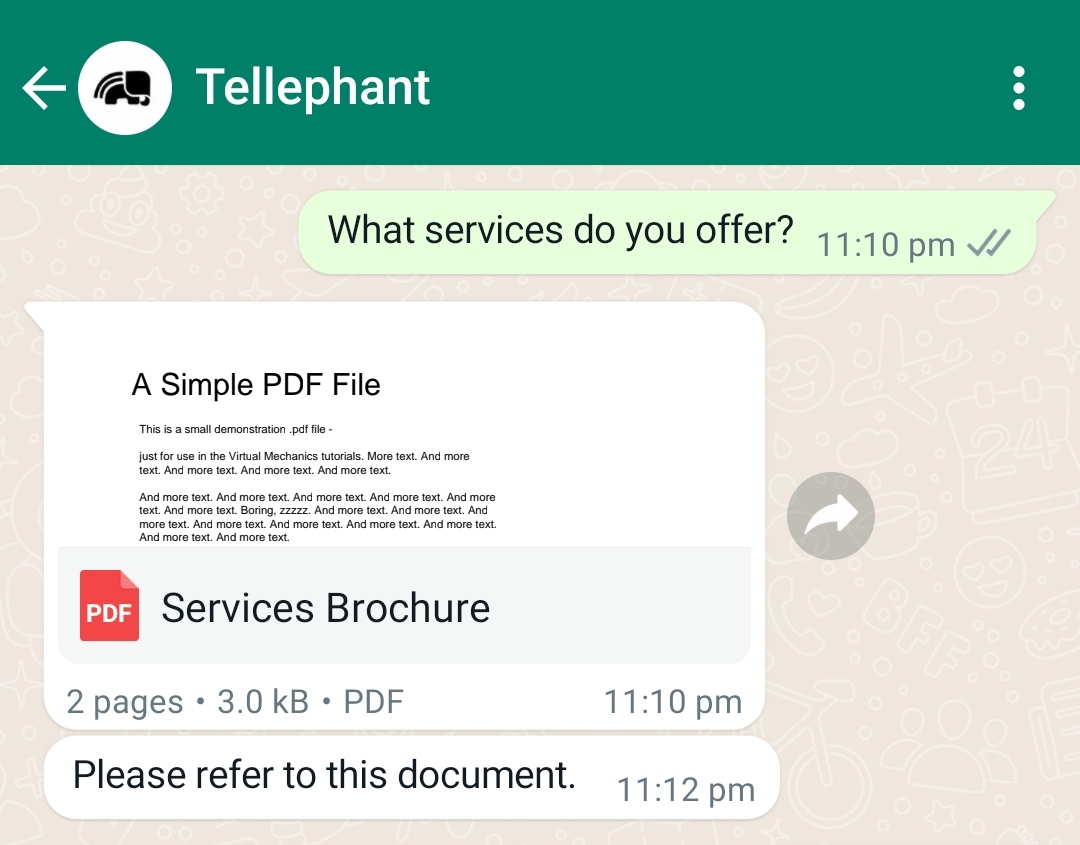
Session Message (STICKER)
Parameter | Type | Status | Description |
---|---|---|---|
contentType |
string | required | Media header for WhatsApp Session message |
type |
String | required | Type of media used |
url |
String | required | Location where the sticker is stored |
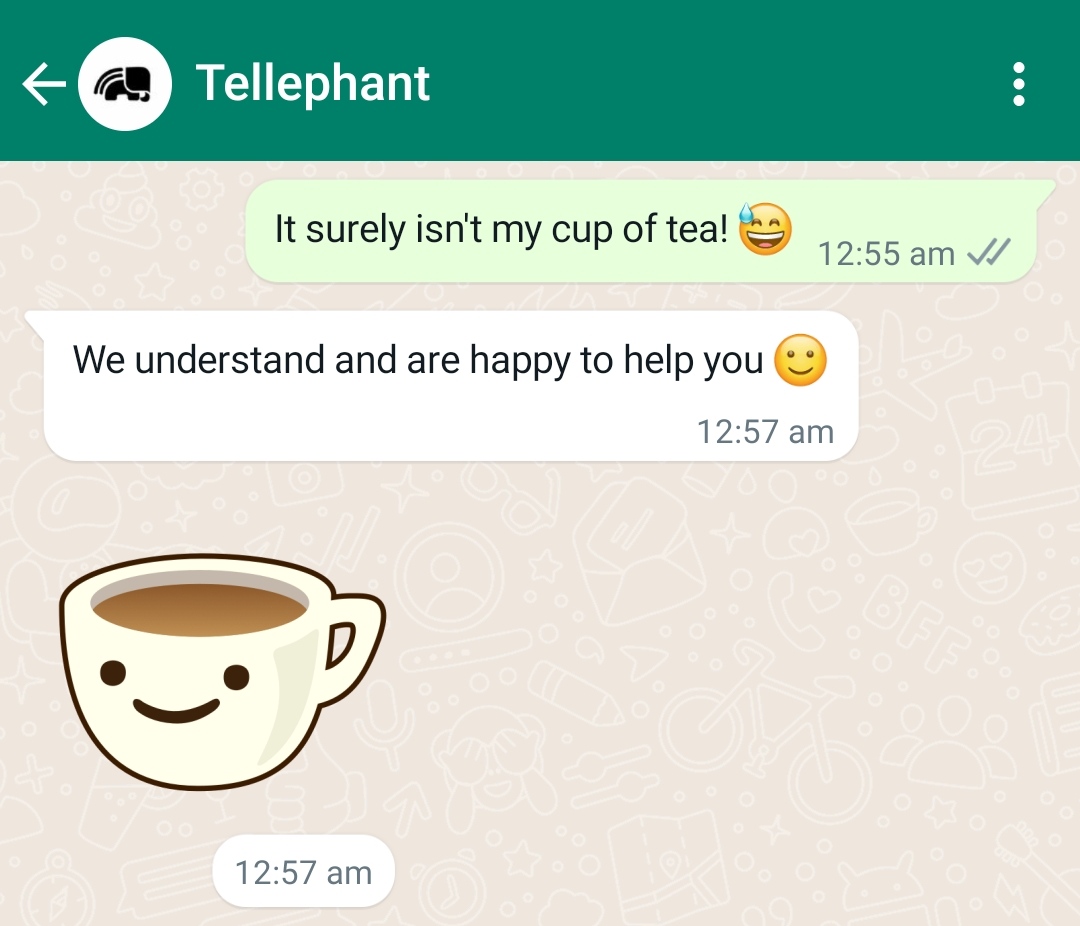
Session Message (VIDEO)
Parameter | Type | Status | Description |
---|---|---|---|
contentType |
string | required | Media header for WhatsApp Session message |
type |
String | required | Type of media used |
url |
String | required | Location where the video is stored |
caption |
String | optional | Text description alongwith |
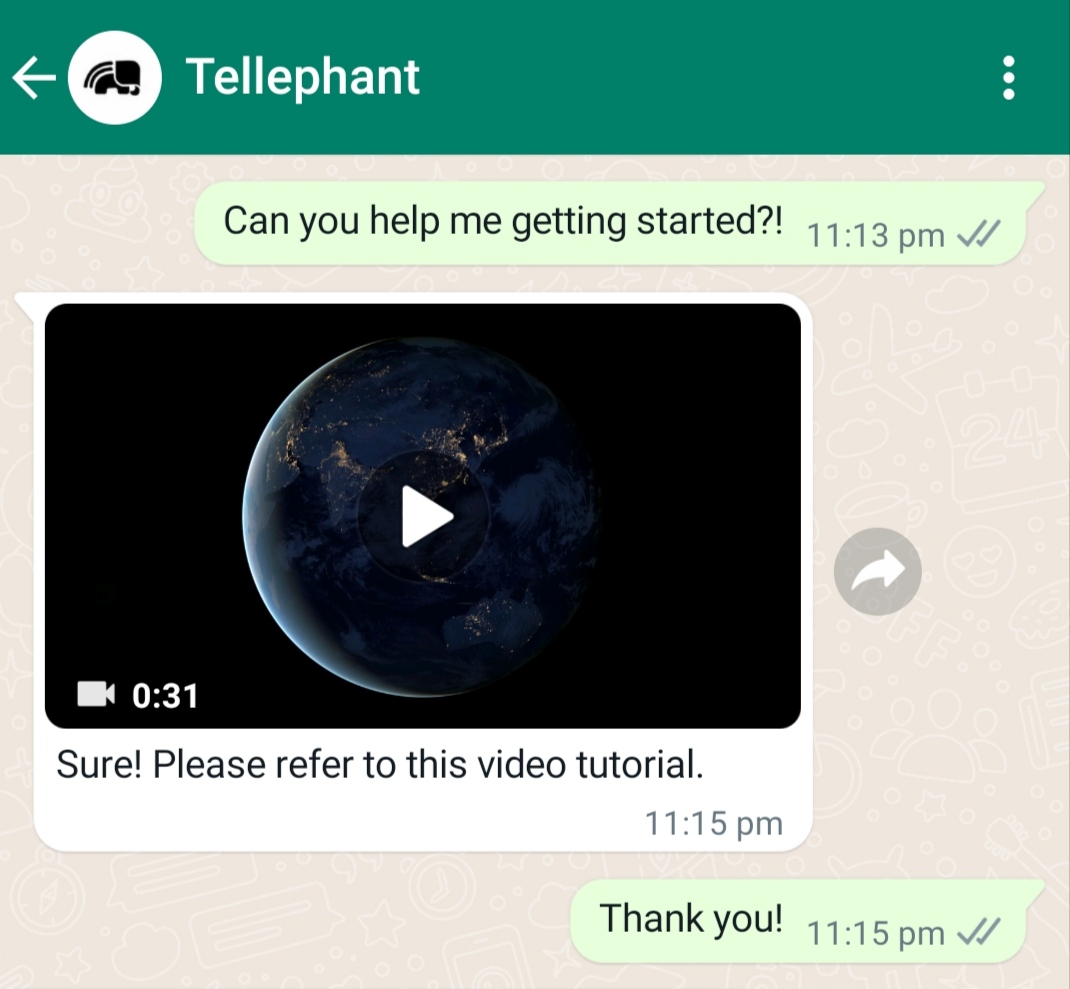
Session Message (AUDIO)
Parameter | Type | Status | Description |
---|---|---|---|
contentType |
string | required | Media header for WhatsApp Session message |
type |
String | required | Type of media used |
url |
String | required | Location where the audio file is stored |
caption |
String | optional | Text description alongwith |
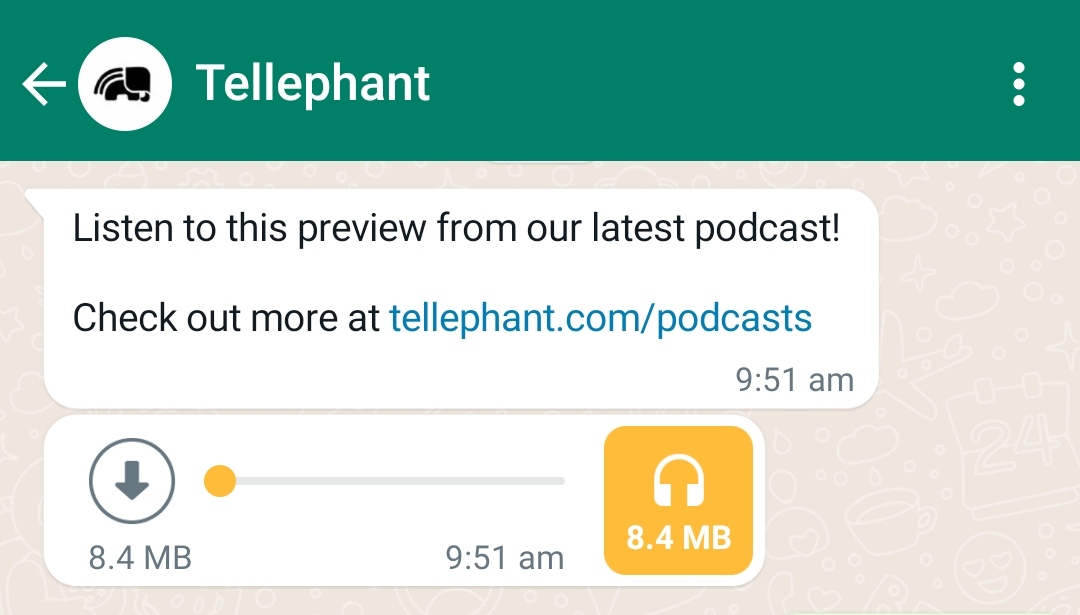
Session Message (LOCATION)
Parameter | Type | Status | Description |
---|---|---|---|
contentType |
string | required | Location header for WhatsApp Session message |
longitude |
number(double) | required | Logitude component of location |
latitude |
number(double) | required | Latitude component of location |
name |
String | required | Name of the location |
address |
String | required | Address of the location |
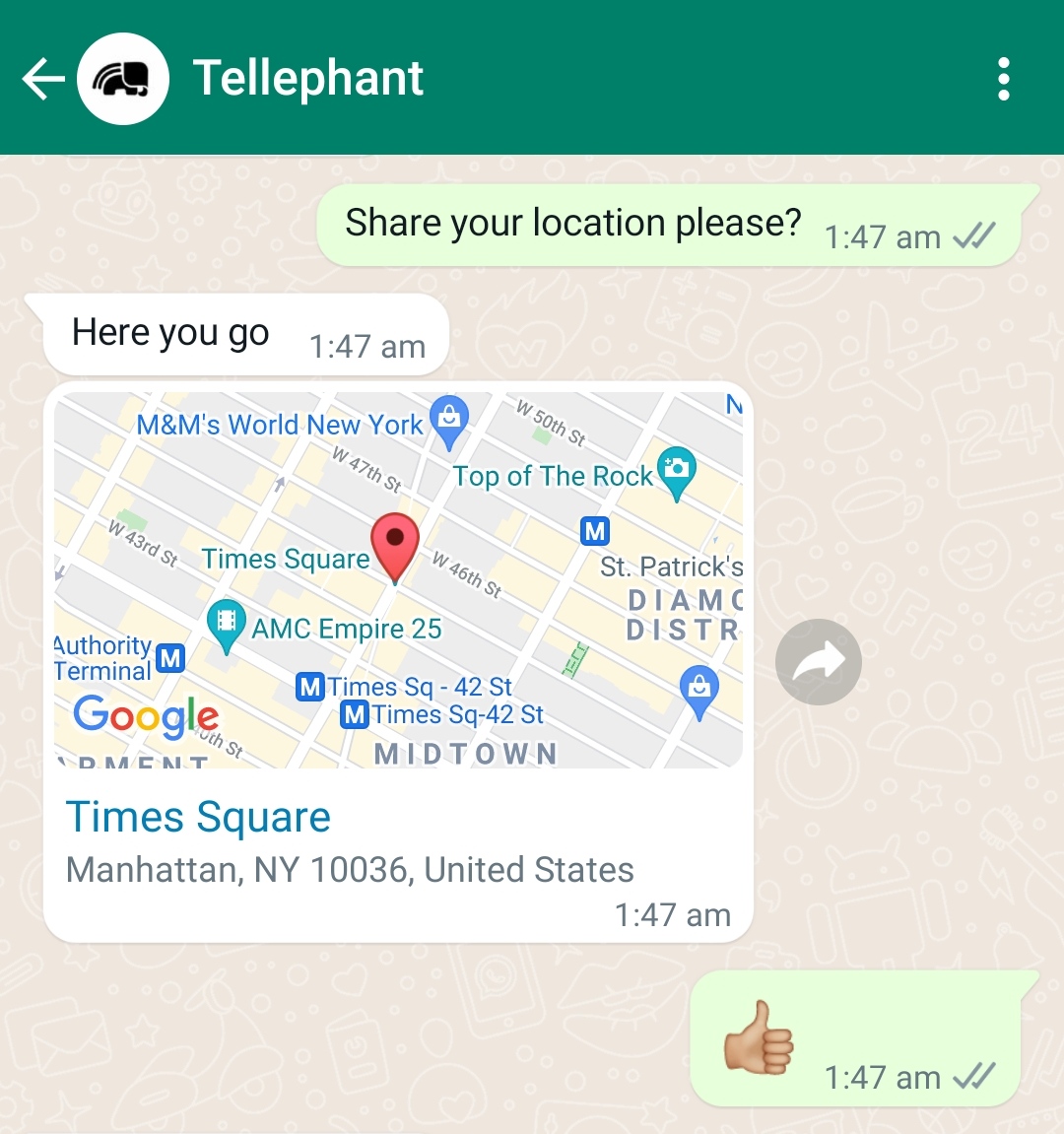
Session Message (CONTACT)
Parameter | Type | Status | Description |
---|---|---|---|
contentType |
string | required | Contact header for WhatsApp Session message |
formattedName |
String | required | Full name of the entity |
firstName |
String | required | First name of the entity |
phone |
String | required | Contact phone number details |
type |
String | required | Type of contact |
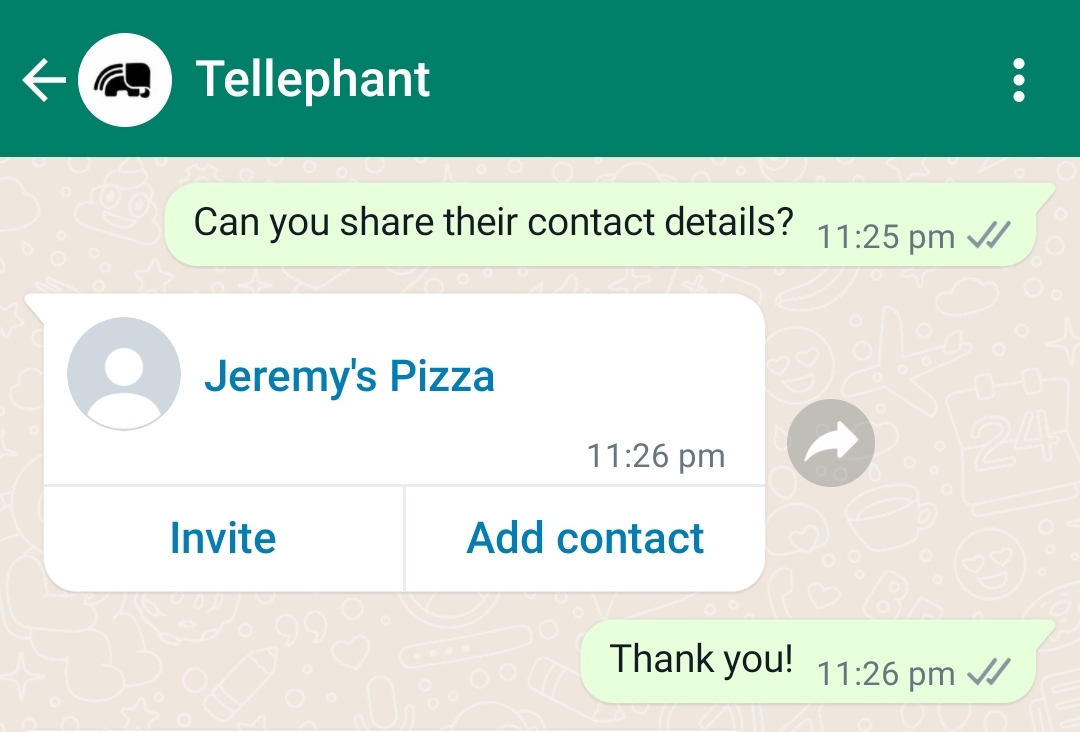
Session Message (Interactive List)
Parameter | Type | Status | Description |
---|---|---|---|
interactive.subType |
string | required | Interactive list header for WhatsApp Session message |
components.header |
object | optional | Message header |
header.text |
string | required | Header text content |
components.body |
object | required | Message body text |
body.text |
string | required | Message body content |
components.footer |
object | optional | Message footer |
footer.text |
string | required | Message footer content |
list.title |
string | optional | Title of the list (Max length: 24 characters) |
list.section |
array | required | Array of section objects (minimum of 1 and maximum of 10) |
section.title |
string | required (if multiple sections) | Title of the section (Max length: 24 characters) |
section.rows |
array | required | Contains a list of rows. |
rows.title |
string | required | row title (Max length: 24 characters) |
rows.payload |
string | required | payload (Max length: 200 characters) |
rows.description |
string | optional | description (Max length: 72 characters) |
Note-
Maximum 10 number of rows allowed. Rows can be wrapped up in sections. A section shall have at least one row.
Payload must be unique for every row.
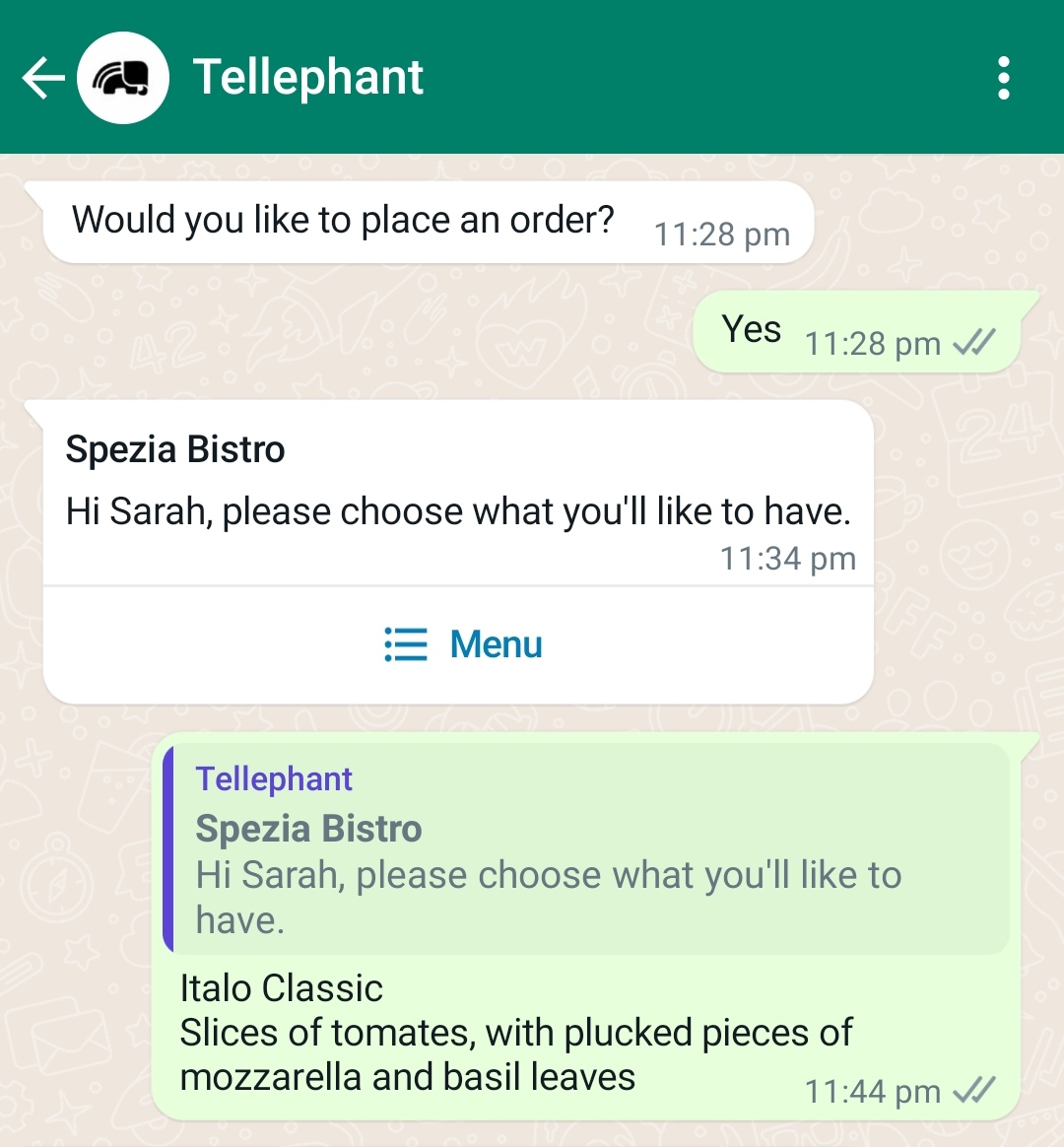
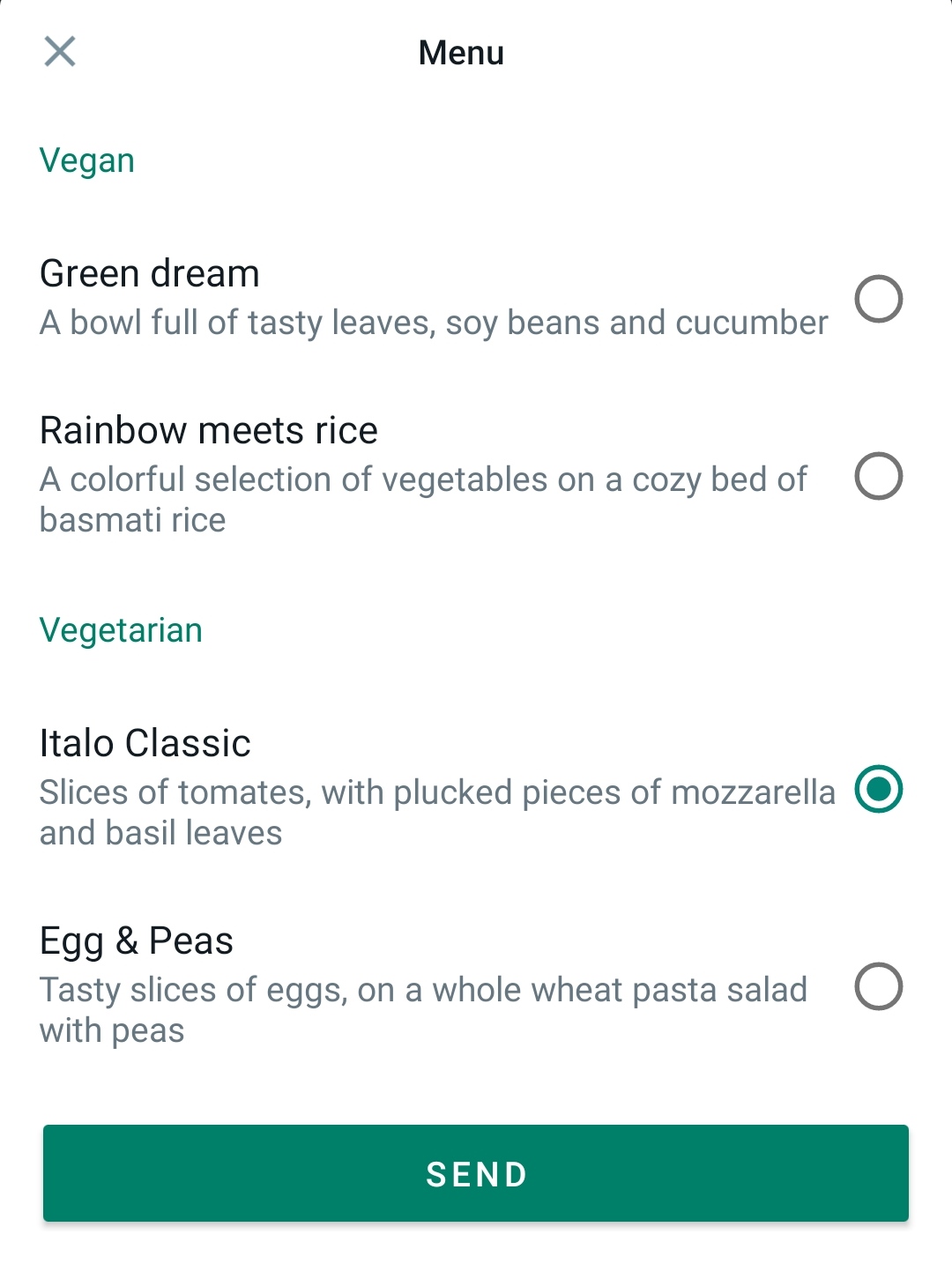
Session Message (Interactive Reply Buttons) - Text Header
Parameter | Type | Status | Description |
---|---|---|---|
interactive.subType |
string | required | Interactive buttons header for WhatsApp Session message |
components.header |
object | optional | Message header |
header.type |
string | required | Type of header |
header.text |
string | required | Header text content |
components.body |
object | required | Message body |
body.text |
string | required | Message body content |
components.footer |
object | optional | Message footer |
footer.text |
string | required | Message footer content |
buttons.type |
string | required | Type of buttons |
reply.title |
string | required | Button title (Max length: 20 characters) |
reply.payload |
string | required | Payload (Max length: 256 characters) |
Note-
Maximum 3 and minimum 1 button shall be included in this request.
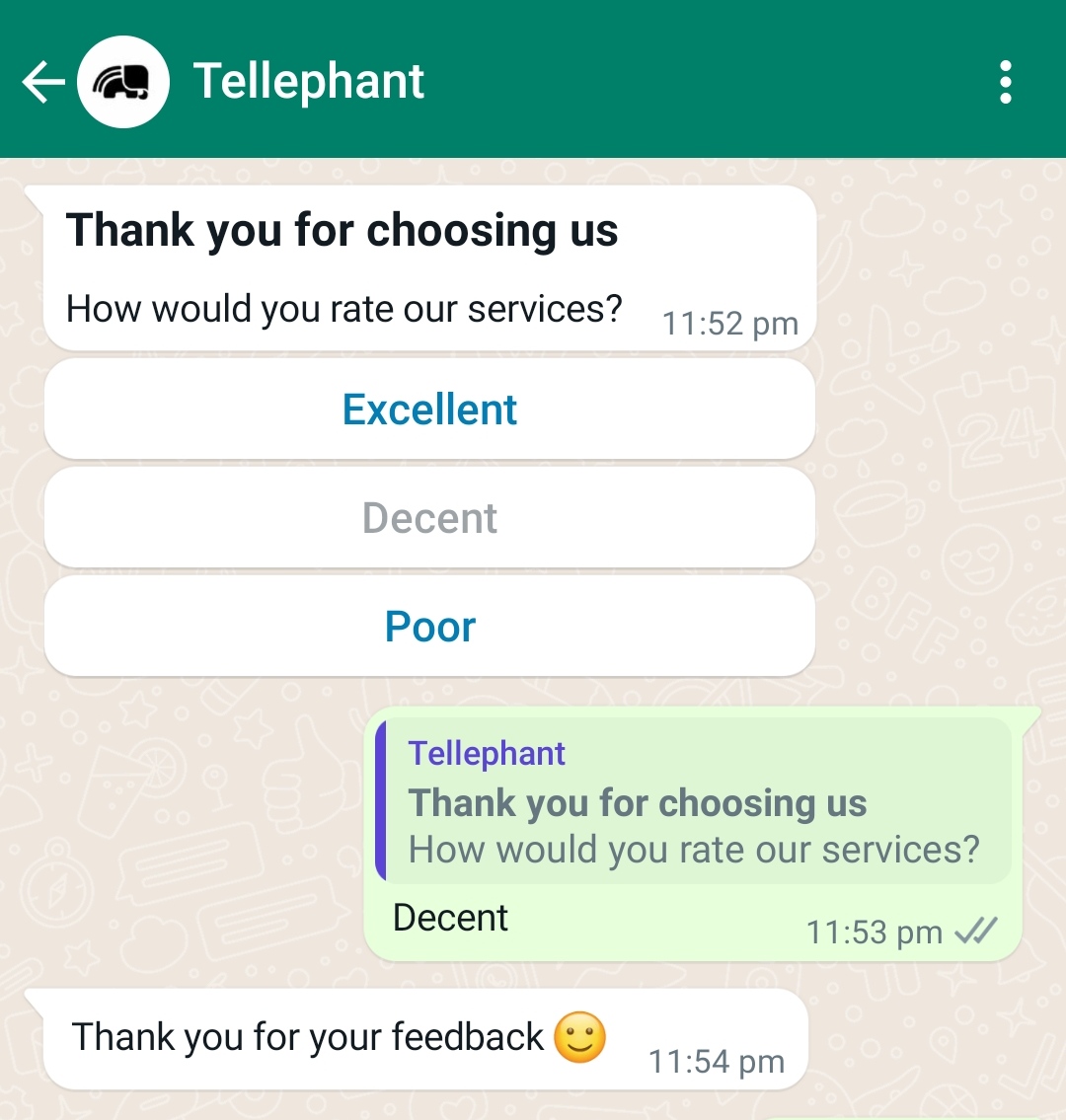
Session Message (Interactive Reply Buttons) - Image Header
Parameter | Type | Status | Description |
---|---|---|---|
interactive.subType |
string | required | Interactive buttons header for WhatsApp Session message |
components.header |
object | optional | Message header |
header.type |
string | required | Type of header |
header.image.url |
string | required | The url of the location where the image is stored |
components.body |
object | required | Message body |
body.text |
string | required | Message body content |
components.footer |
object | optional | Message footer |
footer.text |
string | required | Message footer content |
buttons.type |
string | required | Type of buttons |
reply.title |
string | required | Button title (Max length: 20 characters) |
reply.payload |
string | required | Payload (Max length: 256 characters) |
Note-
Maximum 3 and minimum 1 button shall be included in this request.
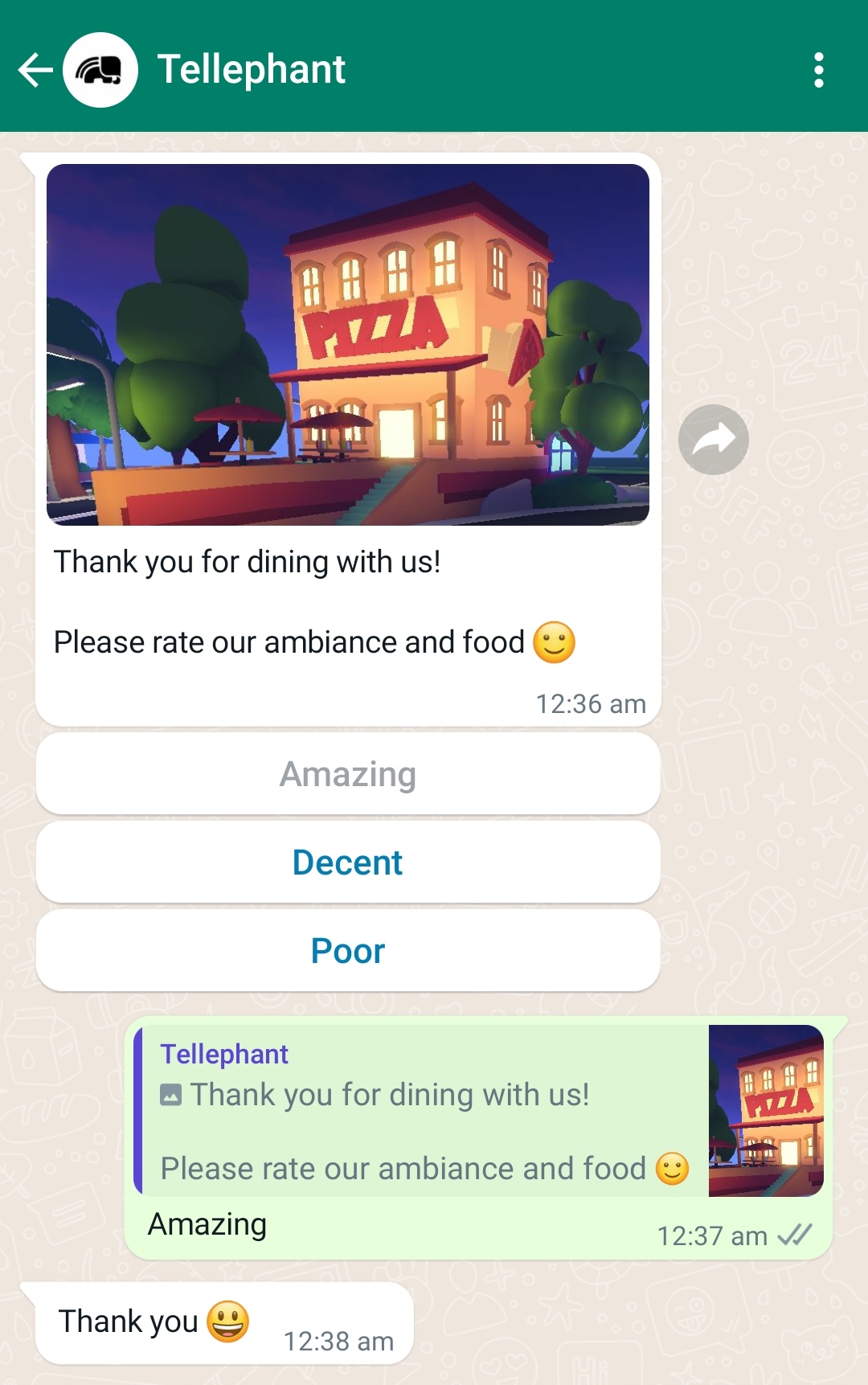
Session Message (Interactive Reply Buttons) - Document Header
Parameter | Type | Status | Description |
---|---|---|---|
interactive.subType |
string | required | Interactive buttons header for WhatsApp Session message |
components.header |
object | optional | Message header |
header.type |
string | required | Type of header |
header.document.url |
string | required | The url of the location where the pdf is stored |
header.document.filename |
string | required | PDF file name |
components.body |
object | required | Message body |
body.text |
string | required | Message body content |
components.footer |
object | optional | Message footer |
footer.text |
string | required | Message footer content |
buttons.type |
string | required | Type of buttons |
reply.title |
string | required | Button title (Max length: 20 characters) |
reply.payload |
string | required | Payload (Max length: 256 characters) |
Note-
Maximum 3 and minimum 1 button shall be included in this request.
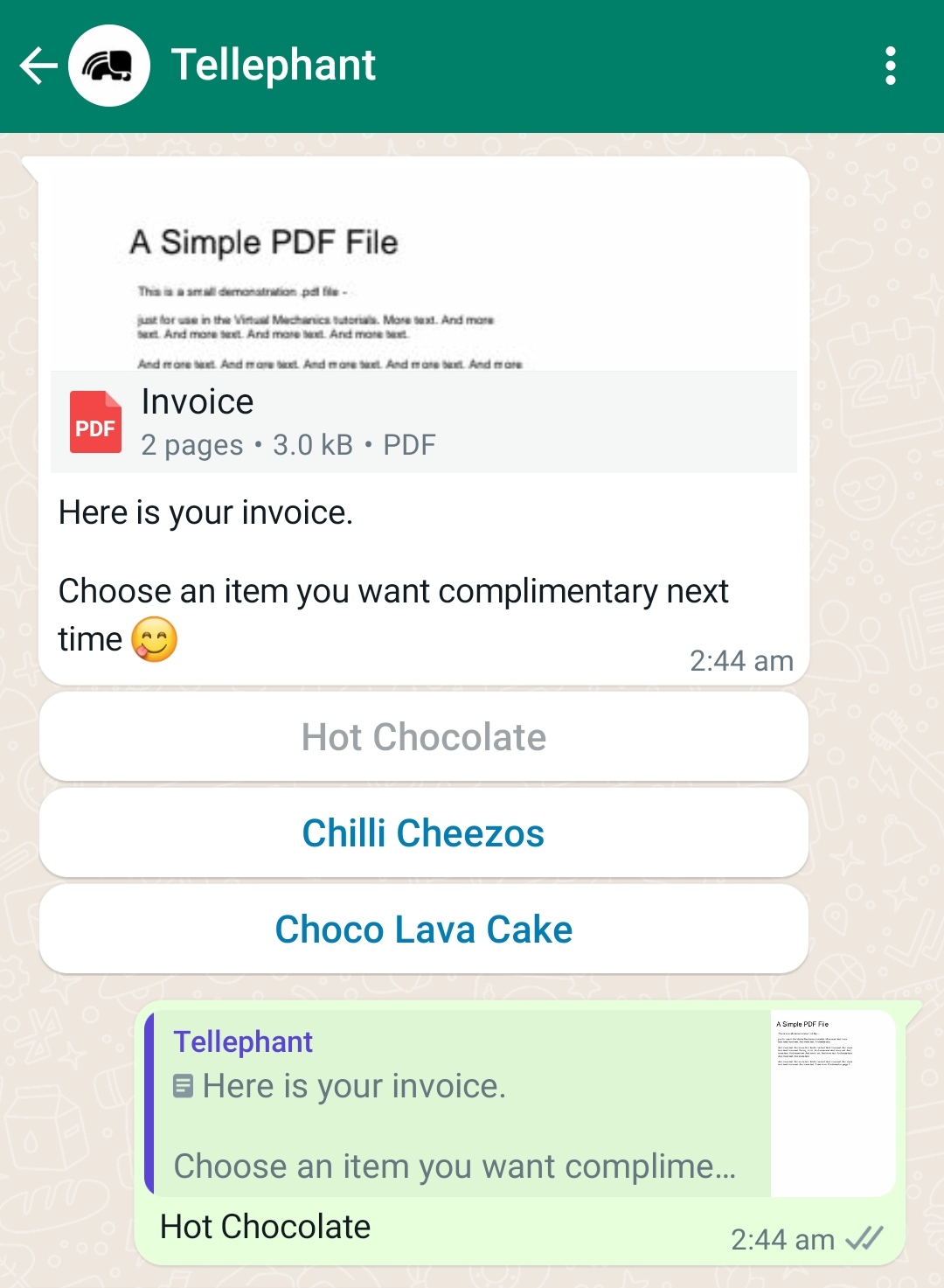
Session Message (Interactive Reply Buttons) - Video Header
Parameter | Type | Status | Description |
---|---|---|---|
interactive.subType |
string | required | Interactive buttons header for WhatsApp Session message |
components.header |
object | optional | Message header |
header.type |
string | required | Type of header |
header.video.url |
string | required | The url of the location where the video is stored |
components.body |
object | required | Message body |
body.text |
string | required | Message body content |
components.footer |
object | optional | Message footer |
footer.text |
string | required | Message footer content |
buttons.type |
string | required | Type of buttons |
reply.title |
string | required | Button title (Max length: 20 characters) |
reply.payload |
string | required | Payload (Max length: 256 characters) |
Note-
Maximum 3 and minimum 1 button shall be included in this request.
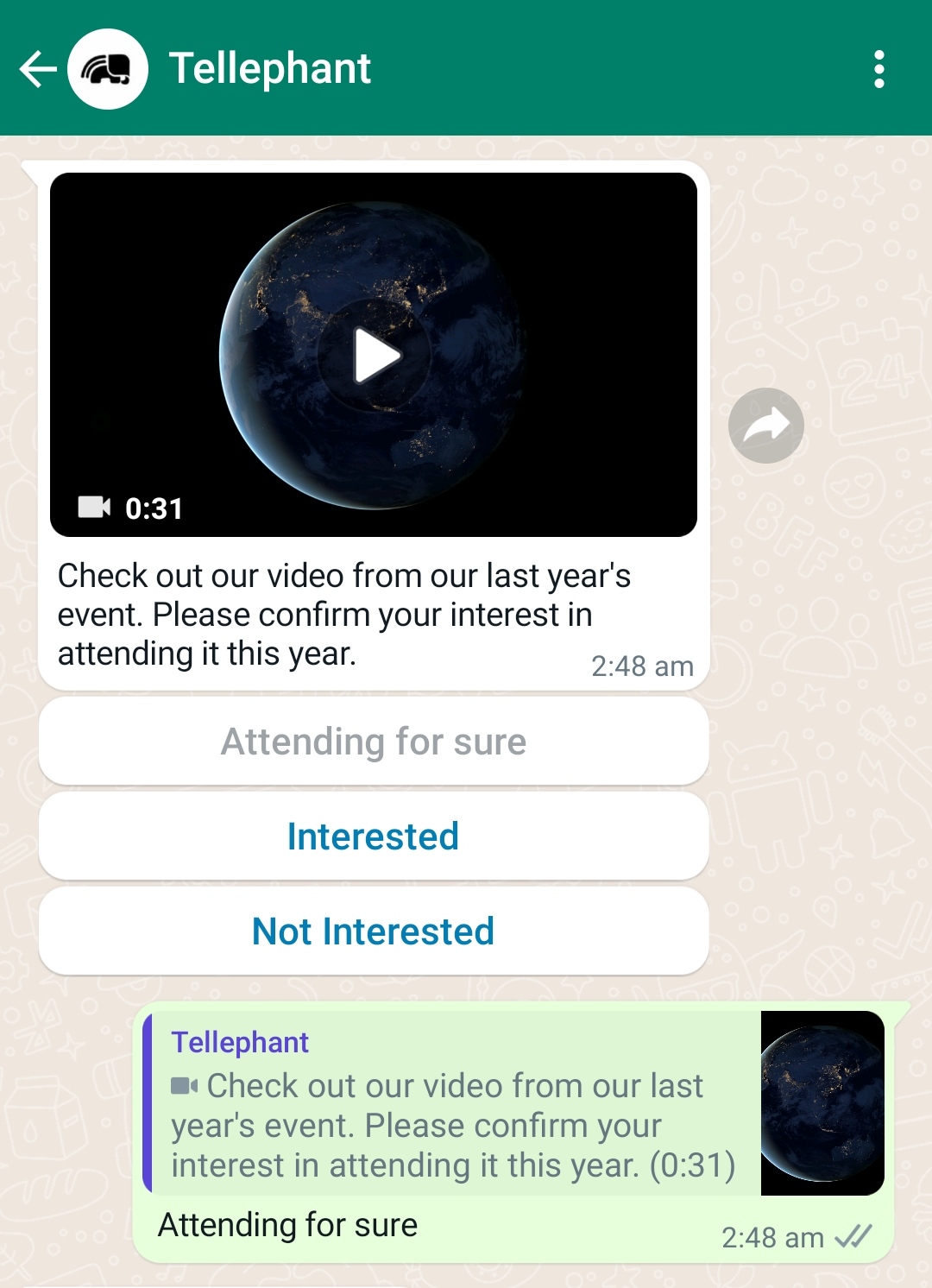
Message History
Message History Example request:
curl --location --request POST 'https://api.tellephant.com/v1/message-history' \
--header 'Content-Type: application/json' \
--data-raw '{
"apikey" : "< apikey >",
"messageId" : "< message id >"
}'
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({"apikey":"< apikey >","messageId":"< message id >"});30
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.tellephant.com/v1/message-history", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.tellephant.com/v1/message-history');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append('{
"apikey" : "< apikey >",
"messageId" : "< message id >"
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import requests
url = "https://api.tellephant.com/v1/message-history"
payload="{\"apikey\" : \"< apikey >\", \"messageId\" : \"< message id >\"}"
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
Example response (200):
[
{
"message_state": "message-accepted",
"happened_at": "2021-09-01T11:04:48+00:00"
},
{
"message_state": "message-delivered",
"happened_at": "2021-09-01T11:04:49+00:00"
},
{
"message_state": "message-read",
"happened_at": "2021-09-01T11:06:32+00:00"
}
]
Example response (401):
{
"error": "Invalid API Key Provided"
}
Example response (404):
{
"error": "Message not found"
}
Check message history via this path.
POST v1/message-history
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
messageId |
String | required | A unique key returned for every message sent |
Note-
The messageId is returned for every HSM/template and session message that is sent.
OTP Service
APIs for using OTP as a Service
Generate OTP
[Request for an OTP.]
Example request:
curl -X POST \
"https://api.tellephant.com/v1/send-otp" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","to":919999999999,"organisation_name":"Aiyo Labs"}'
const url = new URL(
"https://api.tellephant.com/v1/send-otp"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"organisation_name": "Aiyo Labs"
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/send-otp',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'ABCDEFGHIJKLMNOPQRSTUVWXYZ',
'to' => 919999999999,
'organisation_name' => 'Aiyo Labs',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/send-otp'
payload = {
"apikey": "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"to": 919999999999,
"organisation_name": "Aiyo Labs"
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example response (201):
{
"success": true,
"otpId": "CAGHDVFHVFUDUYRFGUFJYR"
}
Example response (422):
{
"status": false,
"error": {
"apikey": [
"The apikey field is required."
],
"to": [
"The to field is required."
],
"organisation_name": [
"The organisation_name field is required."
]
}
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (401):
{
"status": false,
"error": "Please subscribe to addon first"
}
Example response (403):
{
"status": false,
"error": "Balance Low! Please recharge!"
}
HTTP Request
POST v1/send-otp
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
to |
integer | required | to send OTP to. |
organisation_name |
String | required | Name of the organisation. |
Validate the OTP
[Verify the OTP if it's correct]
Example request:
curl -X POST \
"https://api.tellephant.com/v1/validate-otp" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"pi","otpId":"CAGHDVFHVFUDUYRFGUFJYR","otp":123456}'
const url = new URL(
"https://api.tellephant.com/v1/validate-otp"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "pi",
"otpId": "CAGHDVFHVFUDUYRFGUFJYR",
"otp": 123456
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/validate-otp',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'pi',
'otpId' => 'CAGHDVFHVFUDUYRFGUFJYR',
'otp' => 123456,
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/validate-otp'
payload = {
"apikey": "pi",
"otpId": "CAGHDVFHVFUDUYRFGUFJYR",
"otp": 123456
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example response (200):
{
"success": true,
"message": "OTP is valid"
}
Example response (200):
{
"success": false,
"message": "OTP is either expired or already used."
}
Example response (200):
{
"success": false,
"message": "Invalid OtpId Provided."
}
Example response (200):
{
"success": false,
"message": "OTP does not match."
}
Example response (422):
{
"status": false,
"error": {
"apikey": [
"The apikey field is required."
],
"otpId": [
"The otpId field is required."
],
"otp": [
"The otp field is required."
]
}
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
HTTP Request
POST v1/validate-otp
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
otpId |
String | required | OTP ID Received. |
otp |
integer | required | OTP to verify. |
Tags
Create Tags On Platform
Example request:
curl -X POST \
"https://api.tellephant.com/v1/user/tags/create" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey": "",
"data": [
{
"tag_name": "",
"tag_color": ""
},
{
"tag_name": ""
}
]}'
const url = new URL(
"https://api.tellephant.com/v1/user/tags/create"
);
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"apikey": "",
"data": [
{
"tag_name": "",
"tag_color": ""
},
{
"tag_name": ""
}
]
});
var requestOptions = {
method: 'PUT',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.tellephant.com/v1/user/tags/create", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.tellephant.com/v1/user/tags/create');
$request->setRequestMethod('PUT');
$body = new http\Message\Body;
$body->append('{
"apikey" : "",
"data" : [
{
"tag_name" : "",
"tag_color" : ""
},
{
"tag_name" : ""
}
]
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import requests
import requests
import json
url = "https://api.tellephant.com/v1/user/tags/create"
payload = json.dumps({
"apikey": "",
"data": [
{
"tag_name": "",
"tag_color": ""
},
{
"tag_name": ""
}
]
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("PUT", url, headers=headers, data=payload)
print(response.text)
Example response (200):
{
"success": true,
"error": ""
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (401):
{
"success": false,
"error": {
"data.1.tag_name": [
"The data.1.tag_name field is required."
]
}
}
POST v1/user/tags/create
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
string | required | API KEY Provided. |
tag_name |
string | required | Tag name is mandatory |
tag_color |
string | optional | A hexadecimal color code value |
Update Tags for Contacts
Example request:
curl -X POST \
"https://api.tellephant.com/v1/user/tags/update" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","channel":"whatsapp",
"data": [
{
"contact_id": "919999999999",
"tags": [
{
"name": "",
"status": false
},
{
"name": "",
"status": true
}
]
}]
}'
const url = new URL(
"https://api.tellephant.com/v1/user/tags/update"
);
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"apikey": "",
"channel": "whatsapp",
"data": [
{
"contact_id": "919999999999",
"tags": [
{
"name": "",
"status": false
},
{
"name": "",
"status": true
}
]
},
{
"contact_id": "91999999998",
"tags": [
{
"name": "",
"status": true
}
]
}
]
});
var requestOptions = {
method: 'PATCH',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.tellephant.com/v1/user/tags/update", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.tellephant.com/v1/user/tags/update');
$request->setRequestMethod('PATCH');
$body = new http\Message\Body;
$body->append('{
"apikey" : "",
"channel" : "whatsapp",
"data" : [
{
"contact_id" : "919999999999",
"tags" : [
{
"name" : "",
"status" : false
},
{
"name" : "",
"status" : true
}
]
},
{
"contact_id" : "91999999998",
"tags" : [
{
"name" : "",
"status" : true
}
]
}
]
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import requests
import json
url = "https://api.tellephant.com/v1/user/tags/update"
payload = json.dumps({
"apikey": "",
"channel": "whatsapp",
"data": [
{
"contact_id": "919999999999",
"tags": [
{
"name": "",
"status": False
},
{
"name": "",
"status": True
}
]
},
{
"contact_id": "91999999998",
"tags": [
{
"name": "",
"status": True
}
]
}
]
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("PATCH", url, headers=headers, data=payload)
print(response.text)
Example response (200):
{
"success": true,
"error": []
}
Example response (201):
{
"success": true,
"error": {
"91909499994566": "Invalid contact id provided"
}
}
Example response (400):
{
"success": false,
"error": {
"apikey": [
"The apikey field is required."
]
}
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
POST v1/user/tags/update
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
string | required | API KEY Provided. |
channel |
string | required | should be whatsapp |
contact_id |
integer | required | start with country code(w/o special char) |
tag_name |
string | required | Tag name is mandatory |
status |
boolean | required | true or false |
Fetch Contact Info
Example request:
curl -X POST \
"https://api.tellephant.com/v1/user/contacts/919999999999/tags" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ"}'
const url = new URL(
"https://api.tellephant.com/v1/user/contacts/919999999999/tags"
);
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"apikey": ""
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.tellephant.com/v1/user/contacts/919999999999/tags", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.tellephant.com/v1/user/contacts/919999999999/tags');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append('{
"apikey" : ""
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import requests
import json
url = "https://api.tellephant.com/v1/user/contacts/919999999999/tags"
payload = json.dumps({
"apikey": ""
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
Example response (200):
{
"success": true,
"contact_info": {
"tags": [
"test tag"
]
},
"error": ""
}
Example response (400):
{
"success": false,
"error": "Contact not found"
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
POST v1/user/contacts/contact_number/tags
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
string | required | API KEY Provided. |
contact_number |
integer | required | start with country code(w/o special char) |
Fetch Logs
Get Template Logs
Example request:
curl -X POST \
"https://api.tellephant.com/v1/user/logs" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","channel":whatsapp,"log_type":"template","start_date": "15-12-2021", "end_date": "21-12-2021"}
const url = new URL(
"https://api.tellephant.com/v1/user/logs"
);
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "21-04-2022",
"end_date": "21-12-2022",
"log_type": "template"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.tellephant.com/v1/user/logs", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.tellephant.com/v1/user/logs');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append('{
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "15-12-2021",
"end_date": "21-12-2021",
"log_type": "template"
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import requests
import requests
import json
url = "https://api.tellephant.com/v1/user/logs"
payload = json.dumps({
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "15-12-2021",
"end_date": "21-12-2021",
"log_type": "template"
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
Example response (200):
{
"current_page": 1,
"data": [
{
"id": "61c17c6821edbf39d97e254d",
"channel": "whatsapp",
"contact_id": "919999999999",
"text": "Hi",
"message_delivery": {
"accepted": "2021-12-15T12:23:54.020Z",
"delivered": "2021-12-15T12:23:55Z",
"seen": "2021-12-25T15:27:24Z"
},
"message_source": "admin_message",
"message_status": "seen",
"template_id": "account_approval"
}
],
"first_page_url": "https://api.tellephant.com/v1/user/logs?page=1",
"from": 1,
"last_page": 1,
"last_page_url": "https://api.tellephant.com/v1/user/logs?page=1",
"next_page_url": null,
"path": "https://api.tellephant.com/v1/user/logs",
"per_page": 5000,
"prev_page_url": null,
"to": 1,
"total": 1
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (400):
{
"success": false,
"error": {
"log_type": [
"Log Type temp is invalid. Must be one of [outgoing,incoming,template]"
]
}
}
Example response (401):
{
"success": false,
"error": "End Date must be less than or equal to today"
}
POST v1/user/logs
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
string | required | API KEY Provided. |
channel |
string | required | should be whatsapp |
log_type |
string | required | must be one of [outgoing,incoming,template] |
start_date |
string | required | must be less than end date{DD-MM-YYY} |
end_date |
string | required | must be less than or equal to today{DD-MM-YYY} |
Get Incoming Logs
Example request:
curl -X POST \
"https://api.tellephant.com/v1/user/logs" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","channel":whatsapp,"log_type":"incoming","start_date": "01-12-2021", "end_date": "21-12-2021"}
const url = new URL(
"https://api.tellephant.com/v1/user/tags/update"
);
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "21-12-2021",
"end_date": "21-12-2021",
"log_type": "incominsg"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.tellephant.com/v1/user/logs", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.tellephant.com/v1/user/logs');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append('{
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "21-12-2021",
"end_date": "21-12-2021",
"log_type": "incominsg"
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import requests
import json
url = "https://api.tellephant.com/v1/user/logs"
payload = json.dumps({
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "21-12-2021",
"end_date": "21-12-2021",
"log_type": "incominsg"
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
Example response (200):
{
"current_page": 1,
"data": [
{
"id": "61c17c6821edbf39d97e254d",
"channel": "whatsapp",
"contact_id": "919999999999",
"content_type": "text",
"media_url": null,
"message_type": "SESSION",
"sender_name": "tellephant",
"text": "Hi",
"timestamp": 1640070247,
"created_at": "2021-12-21T07:04:08.222000Z"
}
],
"first_page_url": "https://api.tellephant.com/v1/user/logs?page=1",
"from": 1,
"last_page": 1,
"last_page_url": "https://api.tellephant.com/v1/user/logs?page=1",
"next_page_url": null,
"path": "https://api.tellephant.com/v1/user/logs",
"per_page": 5000,
"prev_page_url": null,
"to": 1,
"total": 1
}
Example response (422):
{
"success": false,
"error": {
"log_type": [
"Log Type income is invalid. Must be one of [outgoing,incoming,template]"
]
}
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
POST v1/user/logs
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
string | required | API KEY Provided. |
channel |
string | required | should be whatsapp |
log_type |
string | required | must be one of [outgoing,incoming,template] |
start_date |
string | required | must be less than end date{DD-MM-YYY} |
end_date |
string | required | must be less than or equal to today{DD-MM-YYY} |
Get Outgoing Logs
Example request:
curl -X POST \
"https://api.tellephant.com/v1/user/logs" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","channel":whatsapp,"log_type":"outgoing","start_date": "15-12-2021", "end_date": "21-12-2021"}
const url = new URL(
"https://api.tellephant.com/v1/user/logs"
);
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "22-12-2021",
"end_date": "22-12-2021",
"log_type": "outgoing"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.tellephant.com/v1/user/logs", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.tellephant.com/v1/user/logs');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append('{
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "22-12-2021",
"end_date": "22-12-2021",
"log_type": "outgoing"
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import requests
import json
url = "https://api.tellephant.com/v1/user/logs"
payload = json.dumps({
"apikey": "H4obmi5OpPSQ8aCbLcQ2uA9bypzbY9b9qEad9NwxIuPmXvsRAzXGWIz9Mzbu",
"channel": "whatsapp",
"start_date": "22-12-2021",
"end_date": "22-12-2021",
"log_type": "outgoing"
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
Example response (200):
{
"current_page": 1,
"data": [
{
"id": "61bb2a421c968c43a67cbc38",
"channel": "whatsapp",
"contact_id": "918903460481",
"text": "Testing",
"message_delivery": {
"accepted": "2021-12-16T12:00:02.545Z",
"delivered": "2021-12-16T12:00:04Z",
"seen": "2021-12-16T12:00:47Z"
},
"message_source": "admin_message",
"message_status": "seen",
"message_type": "SESSION",
"template_id": null
}
],
"first_page_url": "https://api.tellephant.com/v1/user/logs?page=1",
"from": 1,
"last_page": 1,
"last_page_url": "https://api.tellephant.com/v1/user/logs?page=1",
"next_page_url": null,
"path": "https://api.tellephant.com/v1/user/logs",
"per_page": 5000,
"prev_page_url": null,
"to": 37,
"total": 37
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (422):
{
"success": false,
"error": {
"start_date": [
"Start Date is not valid. Must be in DD-MM-YY format"
]
}
}
POST v1/user/logs
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
string | required | API KEY Provided. |
channel |
string | required | should be whatsapp |
log_type |
string | required | must be one of [outgoing,incoming,template] |
start_date |
string | required | must be less than end date{DD-MM-YYY} |
end_date |
string | required | must be less than or equal to today{DD-MM-YYY} |
Unsubscribe Users
Update Contact
Example request:
curl -X POST \
"https://api.tellephant.com/v1/user/unsubscribe/update" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"contacts":[
"919999999999"
],
"type":"unsubscribe"}'
const url = new URL(
"https://api.tellephant.com/v1/user/unsubscribe/update"
);
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"apikey": "",
"contacts": [
"919999999999"
],
"type": "blacklist"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.tellephant.com/v1/user/unsubscribe/update", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.tellephant.com/v1/user/unsubscribe/update');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append('{
"apikey": "",
"contacts": [
"919999999999"
],
"type": "blacklist"
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import requests
import requests
import json
url = "https://api.tellephant.com/v1/user/unsubscribe/update"
payload = json.dumps({
"apikey": "",
"contacts": [
"919999999999"
],
"type": "subscribe"
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
response.json()
Example response (200):
{
"success": true,
"message": "Contact(s) successfully unsubscribed"
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
Example response (400):
{
"success": false,
"error": "9999999999 is/are invalid!!"
}
}
Example response (401):
{
"success": false,
"error": {
"type": [
"type subscribed is invalid. Must be in [unsubscribe,subscribe,block,unblock]"
]
}
}
POST v1/user/unsubscribe/update
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
string | required | API KEY Provided. |
contacts |
array | required | start with country code(w/o special char) |
type |
string | required | must be one of [unsubscribe/subscribe/block/unblock] |
Get Contact
Example request:
curl -X POST \
"https://api.tellephant.com/v1/user/unsubscribe" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"ABCDEFGHIJKLMNOPQRSTUVWXYZ","type":"blocked"}'
const url = new URL(
"https://api.tellephant.com/v1/user/unsubscribe"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "pi",
"otpId": "CAGHDVFHVFUDUYRFGUFJYR",
"otp": 123456
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://api.tellephant.com/v1/user/unsubscribe',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'pi',
'otpId' => 'CAGHDVFHVFUDUYRFGUFJYR',
'otp' => 123456,
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://api.tellephant.com/v1/user/unsubscribe'
payload = {
"apikey": "pi",
"otpId": "CAGHDVFHVFUDUYRFGUFJYR",
"otp": 123456
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example response (200):
{
"data": [
"919999999999"
]
}
Example response (400):
{
"success": false,
"error": {
"type": [
"type unsubscribe is invalid. Must be in [all,unsubscribed,blocked]"
]
}
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
POST v1/user/unsubscribe
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
string | required | API KEY Provided. |
type |
string | required | must be one of [all/unsubscribed/blocked] |
Webhook Management
APIs for managing account level information
Get Webhooks
[Get the current webhooks]
Example request:
curl -X POST \
"https://app.tellephant.com/api/v2/user/webhook" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"CAGHDVFHVFUDUYRFGUFJYR"}'
const url = new URL(
"https://app.tellephant.com/api/v2/user/webhook"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "CAGHDVFHVFUDUYRFGUFJYR"
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://app.tellephant.com/api/v2/user/webhook',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'CAGHDVFHVFUDUYRFGUFJYR',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://app.tellephant.com/api/v2/user/webhook'
payload = {
"apikey": "CAGHDVFHVFUDUYRFGUFJYR"
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example response (200):
{
"success": true,
"webhooks": {
"delivery_response": {
"url": "https:\/\/requestbin.com\/r\/XXXXXXXX",
"status": false
},
"incoming_message": {
"url": "https:\/\/requestbin.com\/r\/XXXXXXXXX",
"status": true
}
}
}
Example response (422):
{
"status": false,
"error": {
"apikey": [
"The apikey field is required."
]
}
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
HTTP Request
POST api/v2/user/webhook
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
Update Webhooks
[Update the current webhooks]
Example request:
curl -X POST \
"https://app.tellephant.com/api/v2/user/webhook/update" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d '{"apikey":"CAGHDVFHVFUDUYRFGUFJYR","webhook_type":"delivery_response","webhook_endpoint":"\"https:\/\/requestbin.com\/r\/xxxxxxxx\"","webhook_status":"true"}'
const url = new URL(
"https://app.tellephant.com/api/v2/user/webhook/update"
);
let headers = {
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"apikey": "CAGHDVFHVFUDUYRFGUFJYR",
"webhook_type": "delivery_response",
"webhook_endpoint": "\"https:\/\/requestbin.com\/r\/xxxxxxxx\"",
"webhook_status": "true"
}
fetch(url, {
method: "POST",
headers: headers,
body: body
})
.then(response => response.json())
.then(json => console.log(json));
$client = new \GuzzleHttp\Client();
$response = $client->post(
'https://app.tellephant.com/api/v2/user/webhook/update',
[
'headers' => [
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'apikey' => 'CAGHDVFHVFUDUYRFGUFJYR',
'webhook_type' => 'delivery_response',
'webhook_endpoint' => '"https://requestbin.com/r/xxxxxxxx"',
'webhook_status' => 'true',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
import requests
import json
url = 'https://app.tellephant.com/api/v2/user/webhook/update'
payload = {
"apikey": "CAGHDVFHVFUDUYRFGUFJYR",
"webhook_type": "delivery_response",
"webhook_endpoint": "\"https:\/\/requestbin.com\/r\/xxxxxxxx\"",
"webhook_status": "true"
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
response = requests.request('POST', url, headers=headers, json=payload)
response.json()
Example response (200):
{
"success": true,
"message": "Webhook successfully updated."
}
Example response (422):
{
"status": false,
"error": {
"apikey": [
"The apikey field is required."
],
"webhook_type": [
"The webhook type field is required."
],
"webhook_endpoint": [
"The webhook endpoint field is required."
],
"webhook_status": [
"The webhook status field is required."
]
}
}
Example response (401):
{
"status": false,
"error": "Invalid API Key Provided"
}
HTTP Request
POST api/v2/user/webhook/update
Body Parameters
Parameter | Type | Status | Description |
---|---|---|---|
apikey |
String | required | API KEY Provided. |
webhook_type |
String | required | Webhook Type Provided. |
webhook_endpoint |
Url | required | Webhook Endpoint Provided. |
webhook_status |
Boolean | required | Webhook Status Provided. |
Message Delivery Response
Message Accepted Response
{
"messageId": "60003fae6203e5098b751213",
"status": "accepted",
"timestamp": "2021-01-14T12:57:20.032134Z"
}
Message Delivery Response
{
"messageId": "60003fae6203e5098b751213",
"status": "delivered",
"timestamp": "2021-01-14T12:57:20.253171Z"
}
Message Seen Response
{
"messageId": "60003fae6203e5098b751213",
"status": "seen",
"timestamp": "2021-01-14T12:57:45Z"
}
Note: For every WhatsApp message sent, there are three hits made to your webhook url i.e. (accepted, delivered and seen).
Incoming Payload (Text)
{
"messageId": "600042f62905c2668e678deb",
"from": "Sender_number_with_country_code",
"sender_name": "Sender_name",
"to": "Recipient_number_with_country_code",
"timestamp": "2021-01-14T13:11:17Z",
"content_type": "text",
"text": "Message_body",
"received_at": "2021-01-14T13:11:17Z"
}
Note: for every WhatsApp text message sent by the user to your Official WhatsApp Business Account, the incoming response is as shown above.
Incoming Payload (Image)
{
"messageId": "6000442d78789049501796f9",
"from": "Sender_number_with_country_code",
"sender_name": "Sender_name",
"to": "Recipient_number_with_country_code",
"timestamp": "2021-01-14T13:16:28Z",
"content_type": "image",
"media_url": "file_url",
"received_at": "2021-01-14T13:16:28Z"
}
Note: for every WhatsApp image message sent by the user to your Official WhatsApp Business Account, the incoming response is as shown above.
Incoming Payload (Document)
{
"messageId": "600044a8536544020b0a1346",
"from": "Sender_number_with_country_code",
"sender_name": "Sender_name",
"to": "Recipient_number_with_country_code",
"timestamp": "2021-01-14T13:18:30Z",
"content_type": "document",
"media_url": "file_url",
"filename": "file_name.pdf",
"caption": "file_caption",
"received_at": "2021-01-14T13:18:30Z"
}
Note: for every WhatsApp pdf message sent by the user to your Official WhatsApp Business Account, the incoming response is as shown above.
Incoming Payload (Sticker)
{
"messageId": "600138de6e99f9269c24252d",
"from": "Sender_number_with_country_code",
"sender_name": "Sender_name",
"to": "Recipient_number_with_country_code",
"timestamp": "2021-01-15T06:40:28Z",
"content_type": "sticker",
"media_url": "file_url",
"received_at": "2021-01-15T06:40:28Z"
}
Note: for every WhatsApp sticker message sent by the user to your Official WhatsApp Business Account, the incoming response is as shown above.
Incoming Payload (Video)
{
"messageId": "60013953d0d9562caa25fee2",
"from": "Sender_number_with_country_code",
"sender_name": "Sender_name",
"to": "Recipient_number_with_country_code",
"timestamp": "2021-01-15T06:42:22Z",
"content_type": "video",
"media_url": "file_url",
"received_at": "2021-01-15T06:42:22Z"
}
Note: for every WhatsApp video message sent by the user to your Official WhatsApp Business Account, the incoming response is as shown above.
Incoming Payload (Audio)
{
"messageId": "600139a3650de2794016f889",
"from": "Sender_number_with_country_code",
"sender_name": "Sender_name",
"to": "Recipient_number_with_country_code",
"timestamp": "2021-01-15T06:43:46Z",
"content_type": "voice",
"media_url": "file_url",
"received_at": "2021-01-15T06:43:46Z"
}
Note: for every WhatsApp audio message sent by the user to your Official WhatsApp Business Account, the incoming response is as shown above.
Incoming Payload (Location)
{
"messageId": "600139f1d935a0795a64ef0c",
"from": "Sender_number_with_country_code",
"sender_name": "Sender_name",
"to": "Recipient_number_with_country_code",
"timestamp": "2021-01-15T06:45:04Z",
"content_type": "location",
"location": {
"longitude": 77.0952894,
"latitude": 28.6297149
},
"received_at": "2021-01-15T06:45:04Z"
}
Note: for every WhatsApp location message sent by the user to your Official WhatsApp Business Account, the incoming response is as shown above.
Incoming Payload (Contact)
{
"messageId": "60013a52d0d9562caa25fee6",
"from": "Sender_number_with_country_code",
"sender_name": "Sender_name",
"to": "Recipient_number_with_country_code",
"timestamp": "2021-01-15T06:46:42Z",
"content_type": "contacts",
"contacts": [
{
"addresses": [],
"emails": [],
"ims": [],
"name": {
"firstName": "First_name",
"middleName": "Middle_name",
"lastName": "Last_name",
"formattedName": "Full_Name"
},
"org": {
"company": "Company_Name"
},
"phones": [
{
"phone": "Number_with_country_code",
"type": "Mobile",
"waId": "formated_number"
}
],
"urls": []
}
],
"received_at": "2021-01-15T06:46:42Z"
}
Note: for every WhatsApp contact message sent by the user to your Official WhatsApp Business Account, the incoming response is as shown above.